帮我写一段八叉树点云索引的python代码
时间: 2023-08-28 10:09:22 浏览: 81
以下是一个简单的八叉树点云索引的Python代码实现:
```python
class OctreeNode:
def __init__(self, center, half_width):
self.center = center
self.half_width = half_width
self.children = [None] * 8
self.points = []
class Octree:
def __init__(self, points, max_depth=8, min_points_per_node=5):
self.root = None
self.max_depth = max_depth
self.min_points_per_node = min_points_per_node
self.build_tree(points)
def build_tree(self, points):
min_coords = points.min(axis=0)
max_coords = points.max(axis=0)
center = (min_coords + max_coords) / 2
half_width = np.max(max_coords - center)
self.root = OctreeNode(center, half_width)
for point in points:
self.insert_point(self.root, point, 0)
def insert_point(self, node, point, depth):
if depth == self.max_depth or len(node.points) == self.min_points_per_node:
node.points.append(point)
return
octant = self.get_octant(node, point)
if node.children[octant] is None:
child_center = node.center + self.get_offset(octant) * node.half_width / 2
node.children[octant] = OctreeNode(child_center, node.half_width / 2)
self.insert_point(node.children[octant], point, depth + 1)
def get_octant(self, node, point):
octant = 0
if point[0] > node.center[0]:
octant |= 4
if point[1] > node.center[1]:
octant |= 2
if point[2] > node.center[2]:
octant |= 1
return octant
def get_offset(self, octant):
return np.array([
-1 if octant & 4 else 1,
-1 if octant & 2 else 1,
-1 if octant & 1 else 1
])
def query(self, point, radius):
indices = []
self.query_node(self.root, point, radius, indices)
return indices
def query_node(self, node, point, radius, indices):
if node is None:
return
if np.linalg.norm(point - node.center) > node.half_width + radius:
return
for p in node.points:
if np.linalg.norm(point - p) <= radius:
indices.append(p)
for octant in range(8):
self.query_node(node.children[octant], point, radius, indices)
```
这个实现包括了构建八叉树,插入点,以及查询半径范围内的点。你可以通过创建一个Octree对象来使用这个实现,例如:
```python
import numpy as np
points = np.random.rand(100, 3)
octree = Octree(points)
indices = octree.query(np.array([0.5, 0.5, 0.5]), 0.2)
print(indices)
```
这个例子会在点云中查找距离点(0.5, 0.5, 0.5)半径为0.2的所有点,并打印出它们的索引。
阅读全文
相关推荐
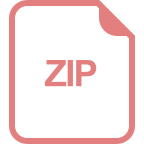

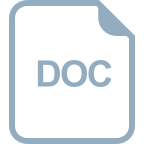
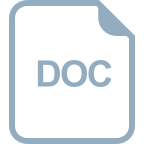









