c++写 创建一个循环队列,初始长度为n 循环队列的入队操作 循环队列的出队操作操作 循环队列的取队头元素操作 求循环队列的长度操作 循环队列的遍历操作
时间: 2023-12-16 11:27:16 浏览: 84
以下是C++实现循环队列的基本操作的代码,包括初始化、入队、出队、取队头元素、遍历输出队列、求队列长度等:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 循环队列的最大长度
class CircularQueue {
private:
int front; // 队头指针
int rear; // 队尾指针
int data[MAXSIZE]; // 队列数组
public:
CircularQueue(int n) { // 初始化队列
front = rear = 0;
for (int i = 0; i < n; i++) {
data[i] = 0;
}
}
bool isEmpty() { // 判断队列是否为空
return front == rear;
}
bool isFull() { // 判断队列是否已满
return (rear + 1) % MAXSIZE == front;
}
int length() { // 求队列长度
return (rear - front + MAXSIZE) % MAXSIZE;
}
bool enqueue(int x) { // 入队操作
if (isFull()) {
return false;
}
data[rear] = x;
rear = (rear + 1) % MAXSIZE;
return true;
}
bool dequeue() { // 出队操作
if (isEmpty()) {
return false; }
front = (front + 1) % MAXSIZE;
return true;
}
int getFront() { // 取队头元素操作
if (isEmpty()) {
return -1;
}
return data[front];
}
void traverse() { // 遍历输出队列
if (isEmpty()) {
cout << "The queue is empty." << endl;
return;
}
cout << "The elements in the queue are: ";
int i = front;
while (i != rear) {
cout << data[i] << " ";
i = (i + 1) % MAXSIZE;
}
cout << endl;
}
};
int main() {
int n = 5; // 循环队列的初始长度为5
CircularQueue q(n);
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.enqueue(4);
q.enqueue(5);
q.traverse(); // 输出:The elements in the queue are: 1 2 3 4 5
q.dequeue();
q.dequeue();
q.traverse(); // 输出:The elements in the queue are: 3 4 5
cout << "The front element is: " << q.getFront() << endl; // 输出:The front element is: 3
cout << "The length of the queue is: " << q.length() << endl; // 输出:The length of the queue is: 3
return 0;
}
```
阅读全文
相关推荐
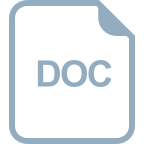
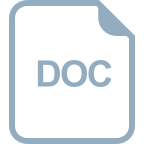
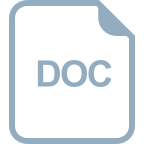














