编写程序,实现循环队列的创建、入队和出队等基本操作算法
时间: 2023-07-16 18:12:04 浏览: 102
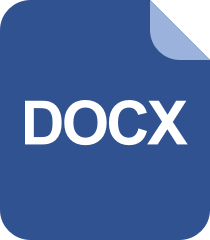
基于TIA博途的循环队列(FIFO)先进先出SCL语言程序算法(V15版本).docx

以下是基于数组实现的循环队列的基本操作算法,包括创建、入队和出队:
1. 创建循环队列
```C++
#define MAXSIZE 100 // 循环队列的最大容量
struct Queue {
int data[MAXSIZE];
int front; // 队列头指针
int rear; // 队列尾指针
};
void InitQueue(Queue &Q) { // 初始化队列
Q.front = Q.rear = 0;
}
```
2. 入队操作
```C++
bool EnQueue(Queue &Q, int x) { // 入队操作
if ((Q.rear + 1) % MAXSIZE == Q.front) { // 队列已满
return false;
}
Q.data[Q.rear] = x;
Q.rear = (Q.rear + 1) % MAXSIZE; // 队尾指针加1
return true;
}
```
3. 出队操作
```C++
bool DeQueue(Queue &Q, int &x) { // 出队操作
if (Q.front == Q.rear) { // 队列为空
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MAXSIZE; // 队头指针加1
return true;
}
```
完整代码如下:
```C++
#include <iostream>
using namespace std;
#define MAXSIZE 100 // 循环队列的最大容量
struct Queue {
int data[MAXSIZE];
int front; // 队列头指针
int rear; // 队列尾指针
};
void InitQueue(Queue &Q) { // 初始化队列
Q.front = Q.rear = 0;
}
bool EnQueue(Queue &Q, int x) { // 入队操作
if ((Q.rear + 1) % MAXSIZE == Q.front) { // 队列已满
return false;
}
Q.data[Q.rear] = x;
Q.rear = (Q.rear + 1) % MAXSIZE; // 队尾指针加1
return true;
}
bool DeQueue(Queue &Q, int &x) { // 出队操作
if (Q.front == Q.rear) { // 队列为空
return false;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MAXSIZE; // 队头指针加1
return true;
}
int main() {
Queue Q;
InitQueue(Q);
EnQueue(Q, 1);
EnQueue(Q, 2);
EnQueue(Q, 3);
int x;
DeQueue(Q, x);
cout << x << endl; // 输出1
DeQueue(Q, x);
cout << x << endl; // 输出2
EnQueue(Q, 4);
EnQueue(Q, 5);
while (DeQueue(Q, x)) {
cout << x << " "; // 输出3 4 5
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
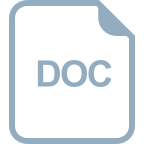
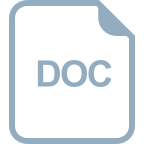













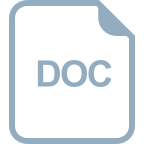
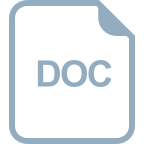