创建一个后台服务,该服务启动后在一个新的线程中持续地以 1 秒间隔更新 通知栏,点击“启动服务”按钮后,服务启动,通知栏上出现图标并按照预设间隔交 替更新;点击“停止服务”按钮后,服务停止,通知栏图标被清除。 交替显示两个不同的图标,
时间: 2023-10-01 16:09:07 浏览: 47
以下是一个简单的示例代码,可以实现你所描述的功能:
```
public class NotificationService extends Service {
private static final int NOTIFICATION_ID = 1;
private static final int UPDATE_INTERVAL = 1000; // 1秒更新一次
private boolean isRunning = false;
private NotificationManager notificationManager;
private int notificationIconIndex = 0;
private int[] notificationIcons = {R.drawable.icon1, R.drawable.icon2};
@Override
public void onCreate() {
super.onCreate();
notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
if (isRunning) {
return START_STICKY;
}
isRunning = true;
new Thread(new Runnable() {
@Override
public void run() {
while (isRunning) {
updateNotification();
try {
Thread.sleep(UPDATE_INTERVAL);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
return START_STICKY;
}
@Override
public void onDestroy() {
super.onDestroy();
isRunning = false;
notificationManager.cancel(NOTIFICATION_ID);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
private void updateNotification() {
NotificationCompat.Builder builder = new NotificationCompat.Builder(this)
.setSmallIcon(notificationIcons[notificationIconIndex])
.setContentTitle("Notification Title")
.setContentText("Notification Content Text")
.setAutoCancel(false);
notificationManager.notify(NOTIFICATION_ID, builder.build());
notificationIconIndex = (notificationIconIndex + 1) % notificationIcons.length;
}
}
```
需要注意的是,这里使用了一个新线程来更新通知栏,因此需要在 `onDestroy` 方法中手动停止这个线程。同时,在 `onStartCommand` 方法中返回 `START_STICKY` 可以让系统在服务被意外终止后自动重启服务。
相关推荐
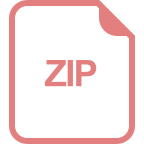
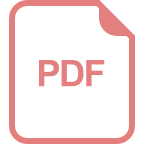
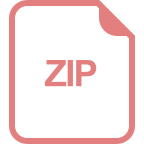














