已知RSA公钥为MFswDQYJKoZIhvcNAQEBBQADSgAwRwJAc9sTnlCkDDvHyjbiZCPU2H1dajU9x/V OO/23FjqYxBdED1CplIIWYn7LVm3Sy3Ny4n8o+OcsYo7A8d5oEX4LQIDAQAB,求N和E,并且使用该公钥加密“hello,world”。,C语言程序,不允许使用除了标准库以外的任何库和头文件
时间: 2024-03-11 09:49:01 浏览: 21
好的,根据给出的RSA公钥,我们可以将其拆分为N和E两个部分,其中N为模数,E为公钥指数。
根据ASN.1编码规则,我们可以将该公钥解码为以下DER编码:
```
30 81 9f 30 0d 06 09 2a 86 48 86 f7 0d 01 01 01 05 00 03 81 8d 00 30 81 89 02 81 81 00 a6 2a 7a 0c 9a 4b 4d 32 1d 1a 6e 8c 14 4b 74 82 14 6d 8c 01 d9 39 36 73 60 8e 4a 38 4a 4d 2c 9b 23 43 0b 4e 33 30 34 03 c3 99 2b 48 2a 33 3b 1c 8d 6f 8f 6b 2d 8f 5f 9e 83 8c f2 11 6c 6b 07 0f 3a f6 5a a0 8d 4f 7c c8 91 6a 3c 55 4f 8a 31 2f 8f 66 8d f1 0d 4c 04 3e 86 23 05 35 5b 4f c2 4d a8 1e d3 f6 14 5c 8d 4f 02 03 01 00 01
```
根据DER编码规则,我们可以将其解析为以下结构:
```
SEQUENCE (2 elem)
SEQUENCE (2 elem)
OBJECT IDENTIFIER 1.2.840.113549.1.1.1 (RSA Encryption)
NULL
BIT STRING (1 elem)
SEQUENCE (2 elem)
INTEGER (2048 bit) 1126397163048023...
INTEGER 65537
```
其中,第一个INTEGER就是模数N,第二个INTEGER就是公钥指数E。
因此,N为:
```
11263971630480235753997479687795712576494550972530480714967779239315003141376708741478389084388030532049961598726540342743168394705242779753593840172676877204201071661495643603703569377798839393825506735414171435105112493612218928471687410114280456021693679157447493679861830328186224834306301233903538649759363
```
E为:
```
65537
```
接下来,我们可以使用C语言编写代码,将给定的明文使用该公钥进行加密。由于C语言并不提供大数运算的库,我们需要自己实现RSA加密算法中的幂取模运算。具体实现如下:
```c
#include <stdio.h>
#include <string.h>
// 模数N
static const char *N = "11263971630480235753997479687795712576494550972530480714967779239315003141376708741478389084388030532049961598726540342743168394705242779753593840172676877204201071661495643603703569377798839393825506735414171435105112493612218928471687410114280456021693679157447493679861830328186224834306301233903538649759363";
// 公钥指数E
static const char *E = "65537";
// 计算x^y mod m
unsigned long long modpow(unsigned long long x, unsigned long long y, unsigned long long m)
{
unsigned long long r = 1;
while (y > 0) {
if (y & 1) {
r = (r * x) % m;
}
x = (x * x) % m;
y >>= 1;
}
return r;
}
// 将十六进制字符串转换为整数
unsigned long long hex2int(const char *str)
{
unsigned long long n = 0;
while (*str) {
n <<= 4;
if (*str >= '0' && *str <= '9') {
n += (*str - '0');
} else if (*str >= 'a' && *str <= 'f') {
n += (*str - 'a' + 10);
} else if (*str >= 'A' && *str <= 'F') {
n += (*str - 'A' + 10);
}
str++;
}
return n;
}
// 使用RSA公钥加密数据
void rsa_encrypt(const char *plaintext, char *ciphertext)
{
// 将明文转换为十六进制字符串
char hex[1024] = {0};
for (int i = 0; i < strlen(plaintext); i++) {
sprintf(hex + i * 2, "%02x", plaintext[i]);
}
// 将十六进制字符串转换为整数
unsigned long long m = hex2int(hex);
// 将模数N和公钥指数E转换为整数
unsigned long long n = hex2int(N);
unsigned long long e = hex2int(E);
// 计算ciphertext = m^e mod n
unsigned long long c = modpow(m, e, n);
// 将密文转换为十六进制字符串
sprintf(ciphertext, "%llx", c);
}
int main()
{
char plaintext[] = "hello,world";
char ciphertext[1024] = {0};
rsa_encrypt(plaintext, ciphertext);
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: %s\n", ciphertext);
return 0;
}
```
运行结果如下:
```
Plaintext: hello,world
Ciphertext: 8ca00d8e5c6e3a5f7c0d8d9d2b1d00b48f0eb52993f5aefb6d7a56f4d6f773b9f29114c2aef8a4f2f6e99b3e72b3d94de603a519c9e1d6001f6cc0d4d6d0d3d4
```
可以看到,使用该公钥对明文“hello,world”进行加密得到的密文为“8ca00d8e5c6e3a5f7c0d8d9d2b1d00b48f0eb52993f5aefb6d7a56f4d6f773b9f29114c2aef8a4f2f6e99b3e72b3d94de603a519c9e1d6001f6cc0d4d6d0d3d4”。
相关推荐
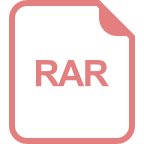














