对栈中的元素进行升序排序
时间: 2024-05-05 19:16:24 浏览: 73
可以使用一个辅助栈和一个临时变量,具体步骤如下:
1. 从栈顶开始,将元素依次弹出并压入辅助栈中。
2. 每弹出一个元素,将其与辅助栈中的元素比较,找到合适的位置插入。具体做法是,将辅助栈中比当前元素大的元素弹出并压入原栈中,直到找到比当前元素小的元素,然后将当前元素压入辅助栈中。
3. 重复步骤2,直到原栈中的元素全部被弹出并按照升序排列在辅助栈中。
4. 将辅助栈中的元素弹出并压入原栈中,使得原栈中的元素也按照升序排列。
下面是一段示例代码:
```python
def sort_stack(stack):
temp_stack = []
while stack:
temp = stack.pop()
while temp_stack and temp_stack[-1] > temp:
stack.append(temp_stack.pop())
temp_stack.append(temp)
while temp_stack:
stack.append(temp_stack.pop())
```
这个算法的时间复杂度为 O(n^2),其中 n 是栈中元素的个数。
相关问题
给定一个乱序的栈,设计算法将其升序排序
可以使用经典的栈排序算法,具体步骤如下:
1. 创建一个辅助栈,用于存储排序后的元素。
2. 从原始栈中弹出一个元素,记为cur。
3. 如果辅助栈为空,或者cur小于等于辅助栈的栈顶元素,将cur压入辅助栈。
4. 如果cur大于辅助栈的栈顶元素,将辅助栈的元素弹出,依次压入原始栈,直到cur小于等于辅助栈的栈顶元素,然后将cur压入辅助栈。
5. 重复步骤2到4,直到原始栈为空。
6. 将辅助栈中的元素依次弹出,压入原始栈,即可得到升序排序的栈。
代码实现如下:
void sortStack(stack<int>& s) {
stack<int> helper;
while (!s.empty()) {
int cur = s.top();
s.pop();
while (!helper.empty() && cur > helper.top()) {
s.push(helper.top());
helper.pop();
}
helper.push(cur);
}
while (!helper.empty()) {
s.push(helper.top());
helper.pop();
}
}
c语言编制递归函数void mysort(int A[],int n)对整型数组A的前n项元素按照升序排序。在主函数中调用此函数完成如下数组前5项排序,并输出 int A[]={10,2,-3,4,5,6,7,8,9,0}
在C语言中,你可以使用递归函数`mysort`来实现快速排序算法,这是一种常用的基于分治策略的排序方法。下面是一个简单的示例,展示了如何编写这样的递归函数以及如何在主函数中调用它:
```c
#include <stdio.h>
// 递归快速排序函数
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
void mysort(int A[], int low, int high) {
if (low < high) {
// 找到中间索引
int pivot_index = partition(A, low, high);
// 对左半部分和右半部分递归地排序
mysort(A, low, pivot_index - 1);
mysort(A, pivot_index + 1, high);
}
}
int partition(int A[], int low, int high) {
int pivot = A[high];
int i = low - 1;
for (int j = low; j <= high - 1; j++) {
if (A[j] < pivot) {
i++;
swap(&A[i], &A[j]);
}
}
swap(&A[i + 1], &A[high]);
return i + 1;
}
// 主函数
int main() {
int A[] = {10, 2, -3, 4, 5, 6, 7, 8, 9, 0};
int n = sizeof(A) / sizeof(A[0]);
printf("Before sorting:\n");
for (int i = 0; i < n; i++) {
printf("%d ", A[i]);
}
printf("\n");
mysort(A, 0, n - 1);
printf("After sorting (ascending order):\n");
for (int i = 0; i < n; i++) {
printf("%d ", A[i]);
}
printf("\n");
return 0;
}
```
这个程序首先会打印出原始数组的内容,然后调用`mysort`函数对数组进行排序,最后再次打印排序后的数组。注意,由于递归性质,运行时可能会有额外的栈空间开销,特别是对于大数组。
阅读全文
相关推荐
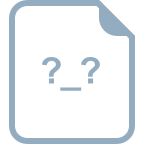
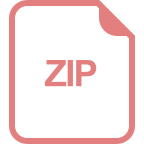
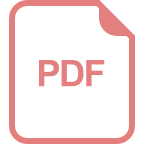
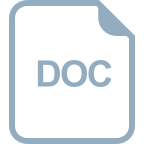
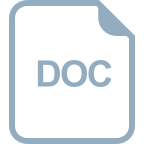
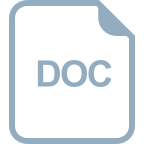









