有两个栈A,B分别储存一个升序数列,现要求编写算法把这两个栈中的数合成一个升序队列
时间: 2024-03-18 08:39:10 浏览: 75
好的,这是一道经典的算法题,可以使用归并排序的思想来解决。
具体实现步骤如下:
1. 新建一个栈C,用于存储合并后的升序队列;
2. 分别从栈A和栈B的顶部取出一个元素,比较大小,将较小的元素压入栈C中,并将其对应的栈指针向下移动一个位置;
3. 重复步骤2,直到其中一个栈为空,此时将另一个非空的栈中的所有元素依次压入栈C中;
4. 最后得到的栈C即为合并后的升序队列。
下面是使用 Python 语言实现的代码示例:
```python
def merge_stack(a, b):
c = []
while a and b:
if a[-1] <= b[-1]:
c.append(a.pop())
else:
c.append(b.pop())
c.extend(reversed(a))
c.extend(reversed(b))
return c[::-1]
```
其中,a和b分别代表两个栈,使用列表来模拟栈的实现,[::-1]表示将列表进行反转,即得到升序队列。
希望能够帮助到你,如果还有其他问题,请继续提问。
相关问题
有两个栈A,B分别储存一个升序数列,现要求编写算法把这两个栈中的数合成一个升序队列用c语言描述
以下是代码实现:
```
#include <stdio.h>
#define MAX_SIZE 100
void mergeStacks(int stackA[], int topA, int stackB[], int topB, int mergedStack[]) {
int i = 0, j = 0, k = 0;
while (i <= topA && j <= topB) {
if (stackA[i] < stackB[j]) {
mergedStack[k++] = stackA[i++];
} else {
mergedStack[k++] = stackB[j++];
}
}
while (i <= topA) {
mergedStack[k++] = stackA[i++];
}
while (j <= topB) {
mergedStack[k++] = stackB[j++];
}
}
int main() {
int stackA[MAX_SIZE], stackB[MAX_SIZE], mergedStack[MAX_SIZE * 2];
int topA = -1, topB = -1;
int n, m;
printf("Enter the size of stack A: ");
scanf("%d", &n);
printf("Enter the elements of stack A in ascending order: ");
for (int i = 0; i < n; i++) {
scanf("%d", &stackA[++topA]);
}
printf("Enter the size of stack B: ");
scanf("%d", &m);
printf("Enter the elements of stack B in ascending order: ");
for (int i = 0; i < m; i++) {
scanf("%d", &stackB[++topB]);
}
mergeStacks(stackA, topA, stackB, topB, mergedStack);
printf("The merged stack in ascending order is: ");
for (int i = 0; i <= topA + topB + 1; i++) {
printf("%d ", mergedStack[i]);
}
return 0;
}
```
在一个升序数列中,插入一个数并保持该数列仍为升序。
在一个升序数列中插入一个数,需要找到该数应该插入的位置,然后将该数插入到该位置。具体步骤如下:
1. 从数列的第一个数开始,依次比较该数和待插入的数的大小,直到找到一个数比待插入的数大或者到达数列的末尾。
2. 如果找到了一个数比待插入的数大,那么待插入的数应该插入到该数的前面,否则待插入的数应该插入到数列的末尾。
3. 将待插入的数插入到相应的位置,然后将该位置后面的数依次向后移动一位。
4. 数列中的数已经按照升序排列,插入一个数后仍然保持升序。
例如,对于升序数列 [1, 3, 5, 7, 9],如果要插入数 4,那么需要比较 4 和 1、3、5、7、9 的大小,发现 4 比 3 大,比 5 小,因此应该插入到 5 的前面,得到新的数列 [1, 3, 4, 5, 7, 9]。
阅读全文
相关推荐
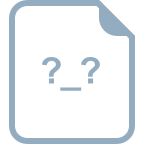
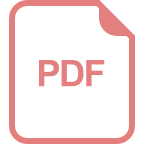
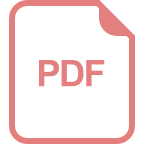
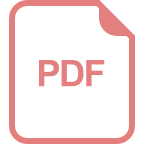
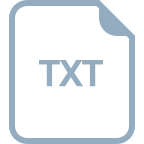
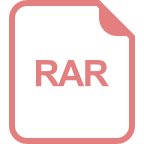
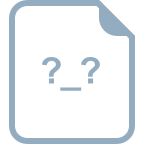




