给定一个字符串,设计一个算法完成对该字符串的一个全排列的输出。比如:字符串 “abc”,输出结果应该是“abc”、“acb”、“bac”、“bca”、“cab” 和 “cba”。 要求:①使用递归思想,编写一个函数 permutationByRecursion,该函数用来生成给定的字符 串的全排列结果。②使用栈数据结构,将①中编写的算法转换成非递归函数,函数名为: permutationByNoRecursion。(利用课堂上讲解的分解 Hanoi 塔的任务方式),该函数所使用的栈 数据结构只能使用自己定义的栈数据结构,不能使用任何语言提供的栈的算法设计
时间: 2023-04-04 11:03:17 浏览: 143
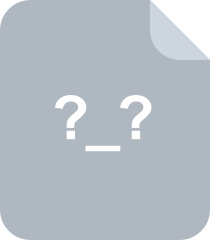
C语言程序设计-对长度为8个字符的字符串,将8个字符按降序排列;例如:原来的字符串为CEAedcab,排序后输出为edcbaE

好的,我会尽力回答你的问题。对于你的第一个问题,我可以回答。以下是我的回答:
①递归算法:
```
void permutationByRecursion(char* str, int start, int end) {
if (start == end) {
printf("%s\n", str);
} else {
for (int i = start; i <= end; i++) {
swap(str + start, str + i);
permutationByRecursion(str, start + 1, end);
swap(str + start, str + i);
}
}
}
```
②非递归算法:
```
void permutationByNoRecursion(char* str, int start, int end) {
Stack* stack = createStack(end - start + 1);
push(stack, start);
int* visited = (int*)calloc(end - start + 1, sizeof(int));
while (!isEmpty(stack)) {
int index = top(stack);
if (visited[index - start] == 0) {
visited[index - start] = 1;
if (index == end) {
printf("%s\n", str);
} else {
for (int i = index; i <= end; i++) {
swap(str + index, str + i);
push(stack, index + 1);
break;
}
}
} else {
visited[index - start] = 0;
pop(stack);
if (!isEmpty(stack)) {
int topIndex = top(stack);
swap(str + topIndex, str + index);
push(stack, topIndex + 1);
}
}
}
free(visited);
destroyStack(stack);
}
```
希望我的回答能够帮到你。
阅读全文
相关推荐
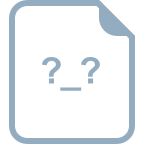


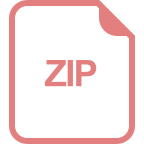
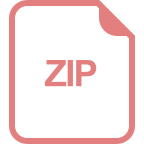
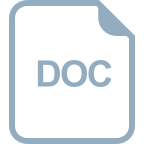
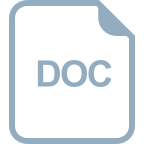








