写一个例子:conftest.py文件中写连接数据库代码,在另一个test0.py文件调用该数据库,写数据库SQL语句如写入数据,查询数据,更新数据,删除数据,删除表
时间: 2024-03-01 12:54:25 浏览: 71
好的,我来为您解答。首先需要明确的是,conftest.py文件是pytest测试框架中的一个钩子函数文件,用于在测试执行前进行一些准备工作,例如连接数据库、读取配置文件等等。而test0.py则是测试用例文件,用于编写具体的测试用例代码。
下面是一个例子:
conftest.py文件中:
```python
import pytest
import pymysql
@pytest.fixture(scope="module")
def db_conn():
conn = pymysql.connect(
host="localhost",
user="testuser",
password="testpass",
database="testdb"
)
yield conn
conn.close()
```
在上述代码中,我们使用了pytest的fixture功能,定义了一个db_conn的fixture,作用域为module,也就是在所有的测试用例执行前都会执行一次。在该fixture中,我们连接了一个名为testdb的MySQL数据库,并返回了连接对象。
test0.py文件中:
```python
def test_insert_data(db_conn):
cursor = db_conn.cursor()
sql = "INSERT INTO user (name, age) VALUES (%s, %s)"
val = ("Alice", 25)
cursor.execute(sql, val)
db_conn.commit()
assert cursor.rowcount == 1
cursor.close()
def test_query_data(db_conn):
cursor = db_conn.cursor()
sql = "SELECT * FROM user WHERE name = %s"
val = ("Alice", )
cursor.execute(sql, val)
result = cursor.fetchone()
assert result is not None
assert result[1] == "Alice"
assert result[2] == 25
cursor.close()
def test_update_data(db_conn):
cursor = db_conn.cursor()
sql = "UPDATE user SET age = %s WHERE name = %s"
val = (26, "Alice")
cursor.execute(sql, val)
db_conn.commit()
assert cursor.rowcount == 1
cursor.close()
def test_delete_data(db_conn):
cursor = db_conn.cursor()
sql = "DELETE FROM user WHERE name = %s"
val = ("Alice", )
cursor.execute(sql, val)
db_conn.commit()
assert cursor.rowcount == 1
cursor.close()
def test_drop_table(db_conn):
cursor = db_conn.cursor()
sql = "DROP TABLE IF EXISTS user"
cursor.execute(sql)
cursor.close()
```
在上述代码中,我们编写了四个测试用例,分别对应插入数据、查询数据、更新数据、删除数据这四种操作。其中,我们都使用了db_conn这个fixture来获取数据库连接对象,并在每个测试用例中创建了游标对象进行SQL语句的执行。在每个测试用例执行完毕后,我们都需要关闭游标对象,确保资源被释放。
最后一个测试用例test_drop_table是用于删除测试用的表user,以便下一次测试时重新创建。这个测试用例执行完毕后,我们也需要关闭游标对象。
阅读全文
相关推荐
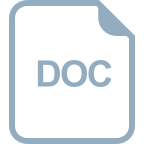
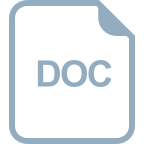
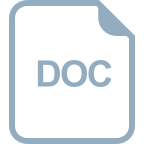




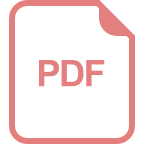
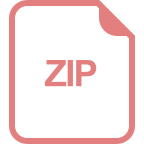
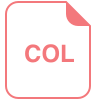
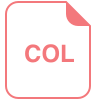
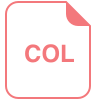






