I want to use the above code in the class. Please give an example
时间: 2023-03-20 15:00:29 浏览: 116
Certainly! Here's an example of how you can use the code in a Python class:
```python
class Calculator:
def __init__(self):
self.result = 0
def add(self, x, y):
self.result = x + y
return self.result
def subtract(self, x, y):
self.result = x - y
return self.result
def multiply(self, x, y):
self.result = x * y
return self.result
def divide(self, x, y):
if y == 0:
raise ValueError("Cannot divide by zero!")
self.result = x / y
return self.result
```
In this example, we've defined a class called `Calculator`. This class has four methods: `add`, `subtract`, `multiply`, and `divide`. Each of these methods takes two arguments (`x` and `y`) and returns the result of the corresponding mathematical operation on those two arguments.
When you create an instance of the `Calculator` class, you can use its methods to perform mathematical operations:
```python
>>> calc = Calculator()
>>> calc.add(2, 3)
5
>>> calc.subtract(5, 2)
3
>>> calc.multiply(4, 6)
24
>>> calc.divide(10, 2)
5.0
```
In this example, we create an instance of the `Calculator` class and store it in the `calc` variable. We then use the `add`, `subtract`, `multiply`, and `divide` methods to perform various mathematical operations. Each method updates the `result` attribute of the `calc` instance, which we can access directly if we want:
```python
>>> calc.result
5.0
>>> calc.add(3, 4)
7
>>> calc.result
7
```
I hope this example helps you understand how to use the code in a class!
相关推荐
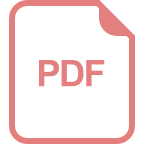
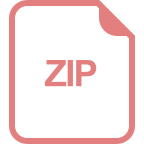
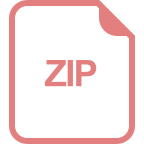















