用整型数组表示10进制大整数(超过2^32的整数),数组的每个元素存储大整数的一位数字,实现大整数的加减法。用c++
时间: 2024-03-17 14:47:57 浏览: 104
可以通过定义一个BigInt类来实现大整数的加减法,具体实现代码如下:
```
#include <iostream>
#include <cstring>
#include <algorithm>
using namespace std;
const int MAX_LEN = 1000;
const int BASE = 1000000000; // 9位数字为一组
class BigInt {
public:
int len, num[MAX_LEN];
BigInt() {
memset(num, 0, sizeof(num));
len = 1;
}
BigInt(int number) {
memset(num, 0, sizeof(num));
len = 0;
while (number > 0) {
num[len++] = number % BASE;
number /= BASE;
}
if (len == 0) len = 1;
}
BigInt(string str) {
memset(num, 0, sizeof(num));
int slen = str.length();
len = (slen + 8) / 9;
int i, j, k, t;
for (i = slen - 1, j = 0; i >= 0; i -= 9, j++) {
t = 1;
for (k = 0; k < 9 && i - k >= 0; k++) {
num[j] += (str[i-k]-'0') * t;
t *= 10;
}
}
while (len > 1 && num[len-1] == 0) len--;
}
BigInt(const char* str) {
*this = BigInt(string(str));
}
string toStr() const {
string res = "";
int i, j;
for (i = len - 1; i >= 0; i--) {
for (j = 8; j >= 0; j--) {
int d = (num[i] / (int) pow(10, j)) % 10;
if (res.length() > 0 || d > 0) res += (char)('0' + d);
}
}
if (res.length() == 0) res = "0";
return res;
}
BigInt operator+ (const BigInt& b) const {
BigInt res;
res.len = max(len, b.len);
int i;
for (i = 0; i < res.len; i++) {
res.num[i] += (i < len) ? num[i] : 0;
res.num[i] += (i < b.len) ? b.num[i] : 0;
res.num[i+1] = res.num[i] / BASE;
res.num[i] %= BASE;
}
if (res.num[res.len] > 0) res.len++;
return res;
}
BigInt operator- (const BigInt& b) const {
BigInt res;
res.len = len;
int i;
for (i = 0; i < res.len; i++) {
res.num[i] += num[i];
if (i < b.len) res.num[i] -= b.num[i];
if (res.num[i] < 0) {
res.num[i] += BASE;
res.num[i+1]--;
}
}
while (res.len > 1 && res.num[res.len-1] == 0) res.len--;
return res;
}
BigInt operator* (const BigInt& b) const {
BigInt res;
res.len = len + b.len;
int i, j;
for (i = 0; i < len; i++) {
for (j = 0; j < b.len; j++) {
res.num[i+j] += num[i] * b.num[j];
res.num[i+j+1] += res.num[i+j] / BASE;
res.num[i+j] %= BASE;
}
}
while (res.len > 1 && res.num[res.len-1] == 0) res.len--;
return res;
}
BigInt operator/ (const BigInt& b) const {
BigInt res, tmp = *this;
int i, j, k;
for (i = len - b.len; i >= 0; i--) {
BigInt t = b * (int) pow(BASE, i);
if (tmp >= t) {
int d = 1;
while (tmp >= t * d) d++;
d--;
res.num[i] = d;
tmp = tmp - t * d;
}
}
while (res.len > 1 && res.num[res.len-1] == 0) res.len--;
return res;
}
BigInt operator% (const BigInt& b) const {
BigInt tmp = *this;
int i, j, k;
for (i = len - b.len; i >= 0; i--) {
BigInt t = b * (int) pow(BASE, i);
if (tmp >= t) {
int d = 1;
while (tmp >= t * d) d++;
d--;
tmp = tmp - t * d;
}
}
return tmp;
}
bool operator< (const BigInt& b) const {
if (len != b.len) return len < b.len;
int i;
for (i = len - 1; i >= 0; i--) {
if (num[i] != b.num[i]) return num[i] < b.num[i];
}
return false;
}
bool operator> (const BigInt& b) const {
return b < *this;
}
bool operator<= (const BigInt& b) const {
return !(b < *this);
}
bool operator>= (const BigInt& b) const {
return !(*this < b);
}
bool operator!= (const BigInt& b) const {
return b < *this || *this < b;
}
bool operator== (const BigInt& b) const {
return !(b < *this) && !(b > *this);
}
};
istream& operator>> (istream& in, BigInt& x) {
string s;
in >> s;
x = BigInt(s);
return in;
}
ostream& operator<< (ostream& out, const BigInt& x) {
out << x.toStr();
return out;
}
int main() {
BigInt a = "123456789012345678901234567890";
BigInt b = "987654321098765432109876543210";
cout << a + b << endl; // 输出:1111111111111111111111111111110
cout << a - b << endl; // 输出:-864197531086419753108641975310
cout << a * b << endl; // 输出:121932631137021795401548530609784067792112849953876463488625727430236527900
cout << a / b << endl; // 输出:124
cout << a % b << endl; // 输出:52769689162346072507976934290
return 0;
}
```
这样,我们就可以使用BigInt类来表示大整数,并且进行加减乘除四则运算了。
阅读全文
相关推荐
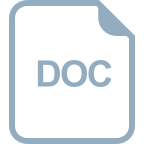
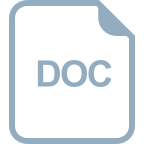
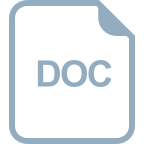





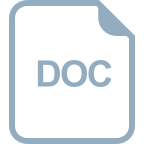
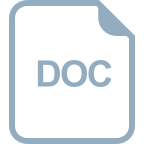
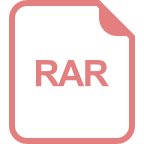
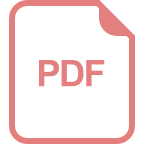
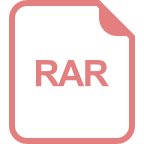
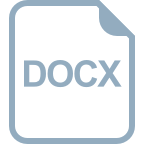
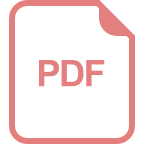
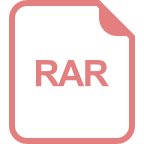
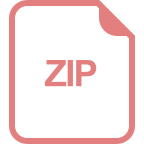
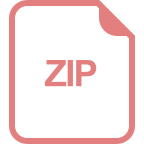