假设一个线性循环队列存储在char c[9]中,初始状态为frrear=0,打印出做完以下操作后队列的头尾指针状态,并给出最终打印c[9]的输出。 1. a, l, l, e, v, i, a, t, e依序入队 2. 出队4个元素 3. o, b, l, i依序入队
时间: 2024-05-10 10:21:43 浏览: 99
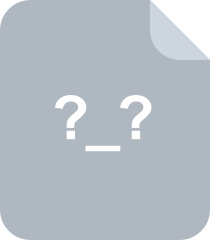
循环队列的初始化、入队、队列长度、取队头元素、出队、遍历等基本操作
操作前队列状态:
```
front = 0
rear = 0
c = [ , , , , , , , , ]
```
操作1:a, l, l, e, v, i, a, t, e依序入队
```
front = 0
rear = 9
c = [a, l, l, e, v, i, a, t, e]
```
操作2:出队4个元素
```
front = 4
rear = 9
c = [ , , , , v, i, a, t, e]
```
操作3:o, b, l, i依序入队
```
front = 4
rear = 1
c = [o, b, l, i, v, i, a, t, e]
```
最终打印c[9]的输出为:
```
v i a t e o b l i
```
阅读全文
相关推荐
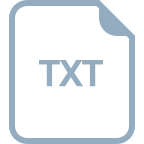
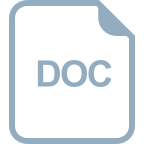
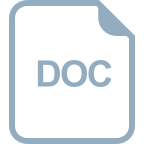

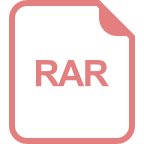
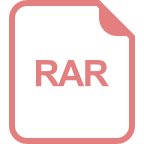
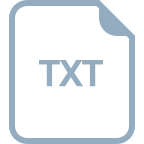
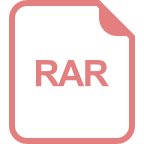
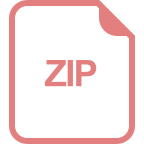
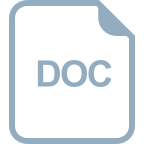
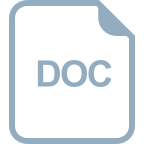
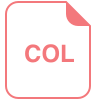
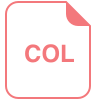



