PySide6 pygragh绘制可旋转的三维坐标轴
时间: 2024-03-27 13:37:21 浏览: 23
要在 PySide6 中使用 pygraph 绘制可旋转的三维坐标轴,可以按照以下步骤进行:
1. 安装 pygraph:使用 pip 命令安装 pygraph,命令如下:
```
pip install pygraph
```
2. 导入必要的库:在 Python 代码中导入必要的库,包括 PySide6 和 pygraph。代码如下:
```python
from PySide6.QtCore import Qt
from PySide6.QtGui import QVector3D
from PySide6.QtWidgets import QApplication, QMainWindow, QGraphicsScene, QGraphicsView
from pygraph import Axis, Graph3D, Grid3D, RotationHandler, ZoomHandler
```
3. 创建图形场景和视图:创建一个 QGraphicsScene 对象和 QGraphicsView 对象来显示 pygraph 绘制的图形。代码如下:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建图形场景和视图
self.scene = QGraphicsScene()
self.view = QGraphicsView(self.scene)
self.view.setRenderHint(QPainter.Antialiasing)
self.view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForAntialiasing, True)
self.view.setOptimizationFlag(QGraphicsView.DontSavePainterState, True)
self.setCentralWidget(self.view)
```
4. 创建 3D 坐标轴和网格:使用 pygraph 中的 Axis 和 Grid3D 类来创建 3D 坐标轴和网格。代码如下:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建图形场景和视图
self.scene = QGraphicsScene()
self.view = QGraphicsView(self.scene)
self.view.setRenderHint(QPainter.Antialiasing)
self.view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForAntialiasing, True)
self.view.setOptimizationFlag(QGraphicsView.DontSavePainterState, True)
self.setCentralWidget(self.view)
# 创建 3D 坐标轴和网格
self.axis = Axis(self.scene, length=10, width=0.2, divisions=10, center=QVector3D(0, 0, 0))
self.grid = Grid3D(self.scene, length=10, width=0.2, divisions=10, center=QVector3D(0, 0, 0))
```
5. 添加旋转和缩放功能:使用 pygraph 中的 RotationHandler 和 ZoomHandler 类来添加旋转和缩放功能。代码如下:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建图形场景和视图
self.scene = QGraphicsScene()
self.view = QGraphicsView(self.scene)
self.view.setRenderHint(QPainter.Antialiasing)
self.view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
self.view.setOptimizationFlag(QGraphicsView.DontAdjustForAntialiasing, True)
self.view.setOptimizationFlag(QGraphicsView.DontSavePainterState, True)
self.setCentralWidget(self.view)
# 创建 3D 坐标轴和网格
self.axis = Axis(self.scene, length=10, width=0.2, divisions=10, center=QVector3D(0, 0, 0))
self.grid = Grid3D(self.scene, length=10, width=0.2, divisions=10, center=QVector3D(0, 0, 0))
# 添加旋转和缩放功能
self.rotation_handler = RotationHandler(self.view)
self.zoom_handler = ZoomHandler(self.view)
```
6. 显示图形:在主程序中显示图形。代码如下:
```python
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec()
```
这样,就可以在 PySide6 中使用 pygraph 绘制可旋转的三维坐标轴了。
相关推荐
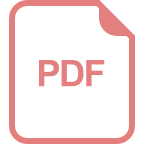














