people[heights[i]]=names[i];
时间: 2023-11-09 13:08:06 浏览: 98
This line of code is assigning the value of the ith index of the 'names' array to the key of the ith index of the 'heights' array in a dictionary called 'people'.
In other words, it is creating a dictionary where the keys are the heights of people and the values are their names. The order of the names and heights in their respective arrays must correspond for this to work correctly.
相关问题
使用kotlin解答这个题目:2418. 按身高排序 提示 简单 83 相关企业 给你一个字符串数组 names ,和一个由 互不相同 的正整数组成的数组 heights 。两个数组的长度均为 n 。 对于每个下标 i,names[i] 和 heights[i] 表示第 i 个人的名字和身高。 请按身高 降序 顺序返回对应的名字数组 names 。 示例 1: 输入:names = ["Mary","John","Emma"], heights = [180,165,170] 输出:["Mary","Emma","John"] 解释:Mary 最高,接着是 Emma 和 John 。 示例 2: 输入:names = ["Alice","Bob","Bob"], heights = [155,185,150] 输出:["Bob","Alice","Bob"] 解释:第一个 Bob 最高,然后是 Alice 和第二个 Bob 。 提示: n == names.length == heights.length 1 <= n <= 103 1 <= names[i].length <= 20 1 <= heights[i] <= 105 names[i] 由大小写英文字母组成 heights 中的所有值互不相同
```kotlin
fun sortNamesByHeight(names: Array<String>, heights: IntArray): Array<String> {
val n = names.size
for (i in 0 until n - 1) {
for (j in i + 1 until n) {
if (heights[i] < heights[j]) {
// 如果 i 的身高比 j 的身高小,则交换 i 和 j 对应的元素
val tmpName = names[i]
val tmpHeight = heights[i]
names[i] = names[j]
heights[i] = heights[j]
names[j] = tmpName
heights[j] = tmpHeight
}
}
}
return names
}
// 测试代码
fun main() {
val names = arrayOf("Mary", "John", "Emma")
val heights = intArrayOf(180, 165, 170)
val sortedNames = sortNamesByHeight(names, heights)
println(sortedNames.contentToString()) // 输出 ["Mary", "Emma", "John"]
}
```
优化下面代码: n = int(input()) heights = list(map(int, input().split())) i = 0 while len(heights) > 1: if i + 1 >= len(heights): a = 0 else: a = i + 1 if heights[i] >= heights[a]: print(heights[a]) del heights[a] if a == 0: i = 0 i = i + 1 if i >= len(heights): i = 0
可以对代码进行如下优化:
```python
n = int(input())
heights = list(map(int, input().split()))
i = 0
while len(heights) > 1:
a = (i + 1) % len(heights)
if heights[i] >= heights[a]:
print(heights[a])
del heights[a]
else:
i += 1
print(*heights, sep='\n')
```
优化说明:
- 使用取模操作 `(i + 1) % len(heights)` 可以简化判断下一个石头的索引,避免使用条件语句。
- 简化了 `if a == 0` 的判断,因为当 `i + 1 >= len(heights)` 时,取模操作 `(i + 1) % len(heights)` 已经保证了 `a` 的值为 0。
- 最后使用 `print(*heights, sep='\n')` 打印剩余的石头高度,避免在循环中多次打印。
请注意,优化代码可能会更加高效,但仍然保持了与原始代码相同的逻辑。
阅读全文
相关推荐
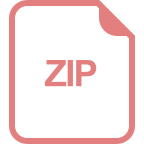
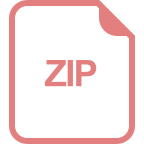













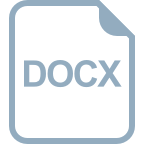
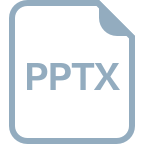