疯狂的打印机 问题描述 分别使用 Thread 和 Runnable 写具有两个同样功能的线程,打印从 1 到 1000(要能区分 是谁打印的)。启动这两个线程观看程序输出。给靠后 start 的线程设置优先级为 8,再 执行一次。并思考结果出现差距的原因。 注:将 2 次执行输出的结果分别保存在 2 个文件中,第一个文件名为:writer.txt, 第二 个文件名为 writer8.txt。
时间: 2024-03-24 11:37:20 浏览: 63
好的,这是一个多线程编程问题,我可以给你提供代码示例和解释。首先,我们看看使用 Thread 的实现:
```java
public class PrinterThread extends Thread {
private int id;
public PrinterThread(int id) {
this.id = id;
}
public void run() {
for (int i = 1; i <= 1000; i++) {
System.out.println("Thread " + id + ": " + i);
}
}
}
public class Main {
public static void main(String[] args) {
PrinterThread t1 = new PrinterThread(1);
PrinterThread t2 = new PrinterThread(2);
t1.start();
t2.setPriority(8);
t2.start();
}
}
```
这里定义了一个 `PrinterThread` 类,继承了 Thread 类,并重写了 run() 方法。在 main() 方法中,我们创建了两个 `PrinterThread` 对象,并分别启动。其中,我们使用 `setPriority()` 方法为第二个线程设置了优先级为 8。这样,我们就可以观察到两个线程的执行情况,并将结果保存到文件中。
接下来,我们来看看使用 Runnable 的实现:
```java
public class PrinterRunnable implements Runnable {
private int id;
public PrinterRunnable(int id) {
this.id = id;
}
public void run() {
for (int i = 1; i <= 1000; i++) {
System.out.println("Runnable " + id + ": " + i);
}
}
}
public class Main {
public static void main(String[] args) {
PrinterRunnable r1 = new PrinterRunnable(1);
PrinterRunnable r2 = new PrinterRunnable(2);
Thread t1 = new Thread(r1);
Thread t2 = new Thread(r2);
t1.start();
t2.setPriority(8);
t2.start();
}
}
```
这里定义了一个 `PrinterRunnable` 类,实现了 Runnable 接口,并重写了 run() 方法。在 main() 方法中,我们创建了两个 `PrinterRunnable` 对象,并将它们分别传入 Thread 的构造函数中创建两个线程。同样地,我们使用 `setPriority()` 方法为第二个线程设置了优先级为 8。
执行以上代码后,你可以看到两个控制台的输出结果,也可以在代码中添加将结果输出到文件的部分。至于优先级的设置,其实并不保证一定会有区别,因为操作系统的调度策略可能会对线程的执行产生影响。但是,一般情况下,我们设置了优先级后,操作系统会更加倾向于调度优先级高的线程,所以可能会出现不同的结果。
阅读全文
相关推荐
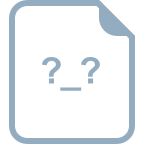
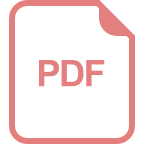
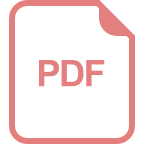



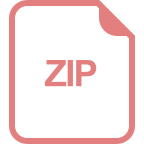
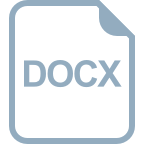
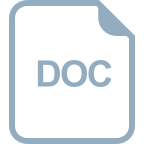
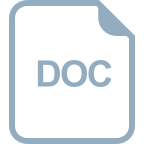
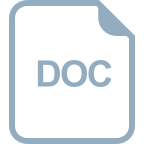
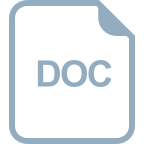
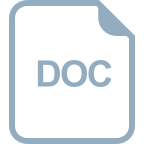
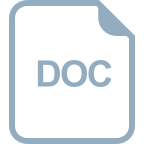
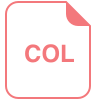



