#include <stdio.h> #include <stdlib.h> #include <pthread.h> #include "jisuan.h" #include "shuru.h" #include "math.h" void *thread_func(void *thread_id); #define NUM_THREADS 2 int main(int argc, char *argv[]) { int rc; pthread_t threads[NUM_THREADS]; int ret; long t; shuru(); // for (t = 0; t < NUM_THREADS; t++) { printf("Creating thread %ld\n", t); rc = pthread_create(&threads[t], NULL, thread_func, (void *) t); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } } for (t = 0; t < NUM_THREADS; t++) { pthread_join(threads[t], NULL); } pthread_exit(NULL); } void *thread_func(void *thread_id) { long tid; tid = (long) thread_id; printf("Thread %ld is running\n", tid); // if(tid == 0) { } if(tid == 1) { double h = 100.0; // 初始高度 double s = 0.0; // 总路程 // 计算落地路程 s = calculate_distance(h); // 输出结果 printf("第%d次落地时,球经过的总路程为:%.6f米\n", num, s); printf("第%d次反弹的高度为:%.6f米\n", num, h / pow(2, num)); } pthread_exit(NULL); }为什么不能正确传递参数num
时间: 2024-03-14 13:45:17 浏览: 25
在这段代码中,变量 num 没有被定义或初始化,所以在调用函数 calculate_distance() 和打印结果时会出现错误。要正确传递参数 num,需要在主函数中定义并初始化它,然后将它作为参数传递给 thread_func() 函数。例如:
```
int num = 10; // 初始化 num
pthread_create(&threads[t], NULL, thread_func, (void *) &num); // 将 num 的地址传递给线程函数
```
然后在 thread_func() 函数中需要使用指针来获取 num 的值,例如:
```
int *p_num = (int *) thread_id; // 将 thread_id 转换为 int 类型的指针
int num = *p_num; // 获取 num 的值
```
这样就可以在线程函数中正确使用 num 变量了。
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#Include<stdio.h>
#include <stdio.h> 是C语言中的一个预处理指令它的作用是将stdio.h头文件包含到当前的源代码文件中。
stdio.h是C语言标准库中的一个头文件,它包含了一些输入输出相关的函数和宏定义。通过包含这个头文件,我们可以使用标准输入输出函数(如printf、scanf等)来进行输入输出操作。
在C语言中,使用#include指令可以将其他头文件包含到当前的源代码文件中,以便在程序中使用头文件中定义的函数、变量或宏等。
需要注意的是,#include指令是在预处理阶段进行处理的,它会将指定的头文件内容直接插入到源代码文件中。因此,当我们在程序中使用了某个头文件时,就需要在程序开始的地方使用#include指令将该头文件包含进来。
希望以上解释对你有帮助。如果还有其他问题,请继续提问。
相关推荐
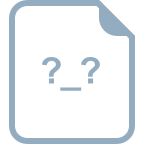
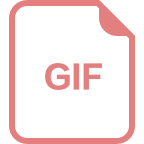
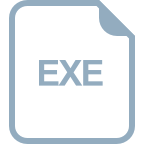
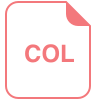
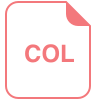











