vue 封装一个步骤表单
时间: 2023-07-01 21:23:45 浏览: 63
好的,我来为你介绍如何使用 Vue 封装一个步骤表单。
1. 创建一个步骤表单组件
首先,我们需要创建一个步骤表单的组件,可以使用 Vue 的单文件组件(.vue)来实现,例如:
```vue
<template>
<div class="step-form">
<div class="step-header">
<div v-for="(step, index) in steps" :key="index" class="step-item">
<div class="step-number">{{ index + 1 }}</div>
<div class="step-title">{{ step.title }}</div>
</div>
</div>
<div class="step-content">
<slot :step="currentStep"></slot>
</div>
<div class="step-footer">
<button @click="prevStep" :disabled="currentStep === 0">上一步</button>
<button @click="nextStep" :disabled="currentStep === steps.length - 1">下一步</button>
<button @click="submitForm" v-if="currentStep === steps.length - 1">提交</button>
</div>
</div>
</template>
<script>
export default {
name: 'StepForm',
props: {
steps: {
type: Array,
required: true
}
},
data() {
return {
currentStep: 0
}
},
methods: {
nextStep() {
this.currentStep++
},
prevStep() {
this.currentStep--
},
submitForm() {
// 提交表单的逻辑
}
}
}
</script>
<style scoped>
.step-header {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 20px;
}
.step-item {
display: flex;
align-items: center;
}
.step-number {
width: 30px;
height: 30px;
border-radius: 50%;
background-color: #ccc;
color: #fff;
display: flex;
justify-content: center;
align-items: center;
margin-right: 10px;
}
.step-title {
font-size: 16px;
font-weight: bold;
}
.step-content {
margin-bottom: 20px;
}
.step-footer {
display: flex;
justify-content: space-between;
}
</style>
```
2. 使用步骤表单组件
在需要使用步骤表单的页面中,可以像使用其他组件一样使用步骤表单组件:
```vue
<template>
<div>
<step-form :steps="steps">
<template v-slot="{ step }">
<div v-if="step === 0">
<!-- 第一步的表单 -->
</div>
<div v-if="step === 1">
<!-- 第二步的表单 -->
</div>
<div v-if="step === 2">
<!-- 第三步的表单 -->
</div>
</template>
</step-form>
</div>
</template>
<script>
import StepForm from './StepForm.vue'
export default {
components: {
StepForm
},
data() {
return {
steps: [
{
title: '第一步'
},
{
title: '第二步'
},
{
title: '第三步'
}
]
}
}
}
</script>
```
在步骤表单组件中,我们使用了插槽(slot)来实现不同步骤对应的表单内容。在使用步骤表单组件时,需要传入一个 steps 属性,该属性是一个包含每个步骤的标题的数组。
相关推荐
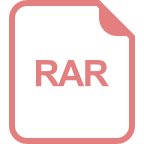
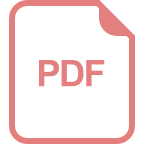














