c++哈希表count
时间: 2023-08-17 14:36:11 浏览: 225
在C++中,哈希表的计数操作可以使用STL中的`std::unordered_map`实现。该容器提供了一个`count`函数,可以用于返回指定键值的元素在容器中出现的次数。例如,以下代码演示了如何使用`std::unordered_map`计算字符串中每个字符出现的次数:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::string str = "hello world";
std::unordered_map<char, int> charCount;
// 计算每个字符出现的次数
for (char c : str) {
charCount[c]++;
}
// 输出结果
for (auto& elem : charCount) {
std::cout << elem.first << ": " << elem.second << std::endl;
}
return 0;
}
```
在上面的代码中,我们先定义了一个`std::unordered_map`对象`charCount`,用于存储每个字符出现的次数。然后,我们遍历字符串中的每个字符,并通过`charCount[c]++`将该字符出现次数加1。最后,我们遍历`charCount`中的每个键值对,输出每个字符及其出现次数。
相关问题
C++ 哈希表 count
在C++中,哈希表是一种数据结构,也称为关联数组或散列表,它通过将键值对映射到内存中的特定位置(索引)来提供高效的查找、插入和删除操作。`std::unordered_map` 或 `std::unordered_set` 是C++标准库提供的内置哈希表容器,用于存储键值对和无序集合。
`count` 是这两个容器的一个成员函数,主要用于统计指定键的数量。例如,在`std::unordered_map`中:
```cpp
std::unordered_map<KeyType, ValueType> myMap;
int count = myMap.count(key); // 如果key存在于map中,返回1;不存在则返回0
```
同样在`std::unordered_set`中:
```cpp
std::unordered_set<ValueType> mySet;
bool found = mySet.count(element); // 如果element在set中,返回true;不在则返回false
```
`count` 函数的时间复杂度通常是 O(1),因为哈希表的查找通常能在常数时间内完成。
c++哈希表count函数
哈希表是一种数据结构,它可以通过散列函数将键映射到存储位置。在C语言中,哈希表通常是使用数组和链表的组合来实现的。
如果你要统计哈希表中某个元素的出现次数,你可以使用count函数来实现。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 10
typedef struct Node {
char key[20];
int value;
struct Node* next;
} Node;
Node* hashTable[SIZE];
// 初始化哈希表
void initializeHashTable() {
for (int i = 0; i < SIZE; ++i) {
hashTable[i] = NULL;
}
}
// 散列函数
int hashFunction(const char* key) {
int sum = 0;
for (int i = 0; i < strlen(key); ++i) {
sum += key[i];
}
return sum % SIZE;
}
// 向哈希表中插入新节点
void insert(const char* key, int value) {
int index = hashFunction(key);
Node* newNode = (Node*)malloc(sizeof(Node));
strcpy(newNode->key, key);
newNode->value = value;
newNode->next = NULL;
if (hashTable[index] == NULL) {
hashTable[index] = newNode;
} else {
Node* currentNode = hashTable[index];
while (currentNode->next != NULL) {
currentNode = currentNode->next;
}
currentNode->next = newNode;
}
}
// 统计元素出现次数
int count(const char* key) {
int index = hashFunction(key);
if (hashTable[index] == NULL) {
return 0;
} else {
int count = 0;
Node* currentNode = hashTable[index];
while (currentNode != NULL) {
if (strcmp(currentNode->key, key) == 0) {
count++;
}
currentNode = currentNode->next;
}
return count;
}
}
int main() {
initializeHashTable();
insert("apple", 1);
insert("banana", 2);
insert("apple", 3);
printf("Count of 'apple': %d\n", count("apple"));
printf("Count of 'banana': %d\n", count("banana"));
return 0;
}
```
在上面的示例代码中,我们定义了一个哈希表结构体`Node`,以及一些相关的操作函数。其中`count`函数用于统计指定键的出现次数。在`main`函数中,我们插入了一些数据,并使用`count`函数来获取指定键的出现次数。输出结果为:
```
Count of 'apple': 2
Count of 'banana': 1
```
希望这个示例代码对你有帮助!如果你有任何问题,请随时提问。
阅读全文
相关推荐

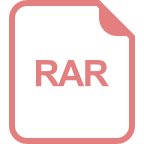
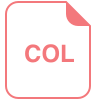










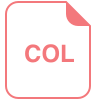
