深入探讨哈希表的冲突解决算法
发布时间: 2024-05-02 07:03:10 阅读量: 6 订阅数: 17 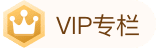
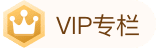
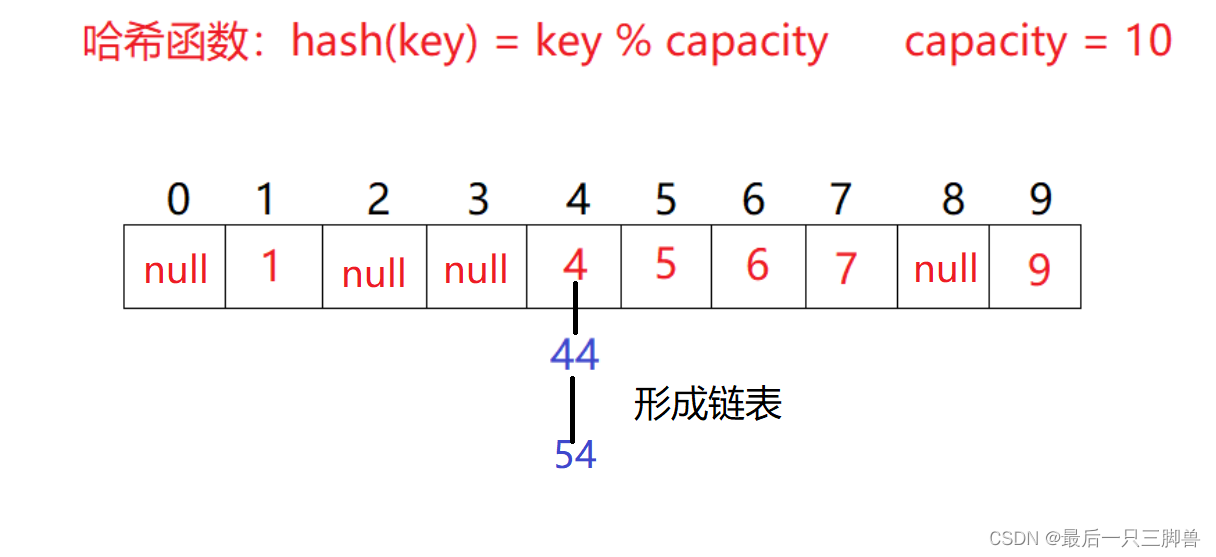
# 1. 哈希表的理论基础
哈希表是一种高效的数据结构,用于快速查找、插入和删除元素。它基于哈希函数的工作原理,该函数将输入数据映射到一个固定大小的数组(称为哈希表)中的唯一索引。
哈希函数将输入数据(称为键)转换为一个哈希值,该哈希值用于确定元素在哈希表中的位置。通过这种方式,哈希表可以将搜索时间复杂度从 O(n)(线性搜索)降低到 O(1)(常数时间)。
哈希表在各种应用中都至关重要,包括集合和映射、缓存和索引,以及哈希查找和哈希表排序等算法。
# 2. 哈希表冲突解决算法
在哈希表中,冲突是指多个键映射到同一个哈希值的情况。为了解决冲突,需要使用冲突解决算法来确定如何存储和检索这些键。
### 2.1 开放寻址法
开放寻址法是一种冲突解决算法,它将冲突的键存储在哈希表中同一位置的连续位置上。
#### 2.1.1 线性探测法
线性探测法是最简单的开放寻址法。当发生冲突时,它将在哈希表中从冲突的位置开始,按顺序查找下一个空位置来存储冲突的键。
```python
def linear_probing(hash_table, key, value):
index = hash(key) % len(hash_table)
while hash_table[index] is not None:
index = (index + 1) % len(hash_table)
hash_table[index] = (key, value)
```
**逻辑分析:**
* `hash(key) % len(hash_table)` 计算键的哈希值并将其映射到哈希表中。
* `while` 循环遍历哈希表,直到找到一个空位置。
* `index = (index + 1) % len(hash_table)` 如果当前位置已占用,则移动到下一个位置。
* `hash_table[index] = (key, value)` 将键值对存储在找到的空位置。
#### 2.1.2 二次探测法
二次探测法是一种改进的开放寻址法,它通过使用二次探测序列来减少冲突。
```python
def quadratic_probing(hash_table, key, value):
index = hash(key) % len(hash_table)
i = 1
while hash_table[index] is not None:
index = (index + i**2) % len(hash_table)
i += 1
hash_table[index] = (key, value)
```
**逻辑分析:**
* `hash(key) % len(hash_table)` 计算键的哈希值并将其映射到哈希表中。
* `while` 循环遍历哈希表,直到找到一个空位置。
* `index = (index + i**2) % len(hash_table)` 使用二次探测序列移动到下一个位置。
* `i += 1` 增加二次探测序列中的步长。
* `hash_table[index] = (key, value)` 将键值对存储在找到的空位置。
#### 2.1.3 双重哈希法
双重哈希法使用两个不同的哈希函数来减少冲突。
```python
def double_hashing(hash_table, key, value):
index1 = hash(key) % len(hash_table)
index2 = hash(key, 2) % len(hash_table)
while hash_table[index1] is not None:
index1 = (index1 + index2) % len(hash_table)
hash_table[index1] = (key, value)
```
**逻辑分析:**
* `hash(key) % len(hash_table)` 计算键的第一个哈希值。
* `hash(key, 2) % len(hash_table)` 计算键的第二个哈希值。
* `while` 循环遍历哈希表,直到找到一个空位置。
* `index1 = (index1 + index2) % len(hash_table)` 使用双重哈希法移动到下一个位置。
* `hash_table[index1] = (key, value)` 将键值对存储在找到的空位置。
### 2.2 链地址法
链地址法是一种冲突解决算法,它将冲突的键存储在哈希表中同一位置的链表中。
#### 2.2.1 单链表法
单链表法使用一个单链表来存储冲突的键。
```python
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.next = None
class HashTable:
def __init__(self):
self.table = [None] * 100
def insert(self, key, value):
index = hash(key) % len(self.table)
if self.table[index] is None:
self.table[index] = Node(key, value)
else:
node = self.table[index]
while node.next is not None:
node = node.next
node.next = Node(key, value)
```
**逻辑分析:**
* `N
0
0
相关推荐





