torch报错AttributeError: 'ModelProto' object has no attribute 'training'
时间: 2023-10-26 13:43:47 浏览: 127
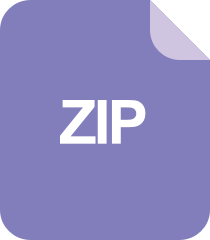
解决Pycharm下载torch报错:OSError: [WinError 126] 找不到指定的模块
这个错误通常是因为你正在尝试使用不支持 `training` 属性的模型,例如从 TensorFlow 转换为 PyTorch 模型时可能会发生这种情况。在这种情况下,你需要手动将模型转换为 PyTorch 模型,并将其放入 PyTorch 的训练模式中。
另外,如果你正在使用的是 PyTorch 模型,则可能是因为你的代码中使用了过时的 API。建议您检查代码中是否有使用过时的函数或方法,并更新为最新版本。
阅读全文
相关推荐
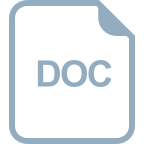
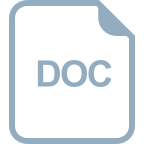










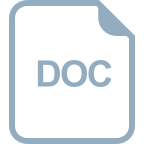
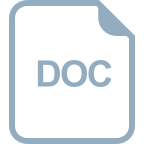