fastapi中创建线程
时间: 2023-09-13 11:07:48 浏览: 219
在 FastAPI 中创建线程可以使用 Python 的 `threading` 模块,具体步骤如下:
1. 导入 `threading` 模块
```python
import threading
```
2. 定义一个函数作为线程的执行体
```python
def worker():
# 执行线程的任务
pass
```
3. 创建一个线程对象,并将执行体函数作为参数传入
```python
t = threading.Thread(target=worker)
```
4. 启动线程
```python
t.start()
```
完整示例代码如下:
```python
import threading
def worker():
# 执行线程的任务
pass
t = threading.Thread(target=worker)
t.start()
```
注意,如果需要在线程中访问 FastAPI 的应用程序实例或请求上下文等对象,需要使用 FastAPI 的 `BackgroundTasks` 或 `Depends` 等功能来传递这些对象。同时,如果在线程中发生异常,FastAPI 的异常处理机制可能无法捕获到该异常,需要在线程内部进行异常处理。
相关问题
fastapi中创建线程传递对象
在 FastAPI 中创建线程并传递对象,可以使用 Python 中的 threading 模块。以下是一种基本的方法:
```python
import threading
from fastapi import FastAPI
app = FastAPI()
class MyThread(threading.Thread):
def __init__(self, obj):
threading.Thread.__init__(self)
self.obj = obj
def run(self):
# 在此处执行需要在新线程中运行的代码,可以使用 self.obj
@app.get("/")
async def root():
my_obj = {"data": "hello world"}
my_thread = MyThread(my_obj)
my_thread.start()
return {"message": "Thread started"}
```
在这个例子中,我们创建了一个自定义的线程类 `MyThread`,它继承自 `threading.Thread`,并且在构造函数中传入了 `obj` 参数。在 `run` 方法中,我们可以执行需要在新线程中运行的代码,并使用传递的 `obj`。在 FastAPI 应用程序中,我们可以在路由处理程序中创建 `MyThread` 实例并启动它,从而在新线程中执行代码。
需要注意的是,如果在新线程中涉及到对 FastAPI 应用程序的请求处理,需要使用异步操作和协程,否则可能会发生阻塞。
fastapi中创建线程传递参数返回值
在 FastAPI 中创建线程并传递参数可以使用 Python 中的 threading 模块,示例如下:
```python
import threading
from fastapi import FastAPI
app = FastAPI()
def my_function(arg1, arg2):
"""
你想要在新线程中执行的函数
"""
# 执行你的代码
result = arg1 + arg2
return result
@app.get("/")
async def root():
# 创建一个新线程并传递参数
thread = threading.Thread(target=my_function, args=(1, 2))
thread.start()
thread.join() # 等待线程执行完毕
result = thread.result # 获取线程执行结果
return {"result": result}
```
在这个示例中,我们定义了一个名为 `my_function` 的函数,它接受两个参数,并返回它们的和。在 FastAPI 中,我们在路由函数上使用 `@app.get("/")` 装饰器,这表示这个路由函数将处理 GET 请求。在路由函数内部,我们使用 `threading.Thread()` 方法创建一个新的线程,并传递参数 `arg1` 和 `arg2` 给 `my_function` 函数。然后我们使用 `thread.start()` 方法启动线程并让它开始执行。
接下来,我们使用 `thread.join()` 方法等待线程执行完毕。这个方法会阻塞当前线程,直到被等待的线程执行完毕。最后,我们使用 `thread.result` 属性获取线程执行结果,并将它作为 JSON 响应返回给客户端。
需要注意的是,如果你在使用线程时需要访问数据库或其他 I/O 操作,最好使用异步方式进行,以避免阻塞主线程。为了实现这一点,你可以使用 Python 的 asyncio 库或第三方库如 aiohttp。
阅读全文
相关推荐

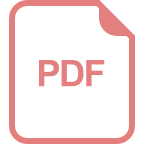
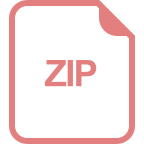
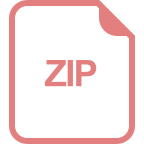
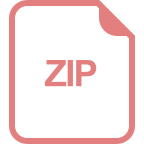
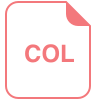
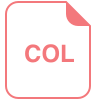
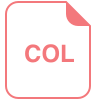
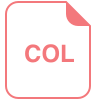
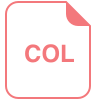
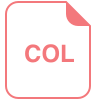



