cpp中string字符串转小写
时间: 2023-07-09 08:43:07 浏览: 50
可以使用标准库中的 `std::transform` 函数和 `std::tolower` 函数来实现将字符串转为小写的功能。具体代码如下:
```cpp
#include <algorithm>
#include <cctype>
#include <string>
std::string toLowerCase(const std::string& str) {
std::string result = str;
std::transform(result.begin(), result.end(), result.begin(),
[](unsigned char c) { return std::tolower(c); });
return result;
}
```
使用示例:
```cpp
std::string str = "Hello World";
std::string lower_str = toLowerCase(str);
// lower_str 的值为 "hello world"
```
相关问题
cpp string中大写字母转小写字母
要将C++中字符串中的大写字母转换为小写字母,可以使用标准库中的 `std::tolower` 函数。具体的实现可以参考以下代码:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
for (auto& c : str) {
if (std::isupper(c)) {
c = std::tolower(c);
}
}
std::cout << str << std::endl; // 输出:hello, world!
return 0;
}
```
在上面的代码中,遍历字符串中的每个字符,如果是大写字母,则使用 `std::tolower` 函数将其转换为小写字母。最后输出转换后的字符串即可。值得注意的是,`std::tolower` 函数只能处理一个字符,因此需要在循环中对每个字符进行转换。
c++ string类 字符串小写
你可以使用 C++ 标准库中的 `transform` 算法和 `tolower` 函数来将字符串中的所有字符转换为小写。以下是一个示例代码:
```cpp
#include <algorithm>
#include <cctype>
#include <string>
int main() {
std::string str = "Hello World!";
std::transform(str.begin(), str.end(), str.begin(),
[](unsigned char c){ return std::tolower(c); });
// 现在 str 中的内容变为 "hello world!"
return 0;
}
```
在这个代码中,`transform` 算法会遍历字符串 `str` 中的每个字符,并将其作为参数传递给 lambda 函数。该 lambda 函数使用 `tolower` 函数将字符转换为小写,并返回转换后的字符。`transform` 算法会将返回值写入到 `str` 中原来的位置,从而实现将整个字符串转换为小写。
相关推荐
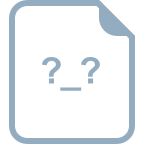
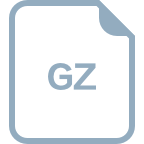












