C语言中用两种算法统计一行文本的单词个数,并展示完整代码
时间: 2023-09-21 15:06:02 浏览: 119
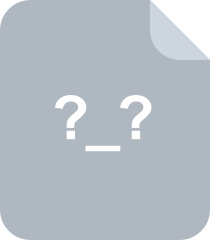
198 单词个数统计 C语言源代码文件
### 回答1:
可以使用哈希表和数组两种算法来统计一行文本中的单词个数,下面是使用哈希表的完整代码:#include<stdio.h>
#include<string.h>
#include<stdlib.h>
#define MAX_LINE_LEN 1000int main(){
char line_str[MAX_LINE_LEN];
int word_count = 0;
char *word;
int i;
int *hash_table; // 分配哈希表的空间
hash_table = (int*)malloc(sizeof(int)*26); // 初始化哈希表
for(i = 0; i < 26; i++)
hash_table[i] = 0; // 读取一行文本
gets(line_str); // 将文本字符串按空格分割
word = strtok(line_str, " "); // 遍历分割后的字符串
while(word != NULL) {
// 将字符串转换为小写字母
for(i = 0; i < strlen(word); i++)
word[i] = tolower(word[i]); // 将单词标识到哈希表中,如果没有标识到,就将单词计数器加1
if(hash_table[word[0]-'a'] == 0){
word_count++;
hash_table[word[0]-'a'] = 1;
} // 指向下一个单词
word = strtok(NULL, " ");
}
printf("单词个数为: %d\n", word_count); // 释放哈希表空间
free(hash_table); return 0;
}
### 回答2:
在C语言中,我们可以使用两种算法分别来统计一行文本的单词个数。下面分别介绍这两种算法,并展示完整的代码。
算法一:遍历法
这种算法通过遍历字符串中的每个字符,当遇到非空字符且其前一个字符为空字符时,就说明遇到了一个单词的起始位置,然后递增计数器统计单词个数。
以下是算法一的完整代码示例:
```c
#include <stdio.h>
#include <stdbool.h>
int countWords(char* str) {
int count = 0;
bool isWord = false;
while (*str) {
if (*str != ' ' && !isWord) {
isWord = true;
count++;
}
else if (*str == ' ') {
isWord = false;
}
str++;
}
return count;
}
int main() {
char str[] = "Hello World! This is a test.";
int wordCount = countWords(str);
printf("Number of words: %d\n", wordCount);
return 0;
}
```
算法二:分割法
这种算法利用C语言中的字符串分割函数strtok来将文本分割成单词,并统计分割得到的单词个数。
以下是算法二的完整代码示例:
```c
#include <stdio.h>
#include <string.h>
int countWords(char* str) {
int count = 0;
char* word = strtok(str, " ");
while (word) {
count++;
word = strtok(NULL, " ");
}
return count;
}
int main() {
char str[] = "Hello World! This is a test.";
int wordCount = countWords(str);
printf("Number of words: %d\n", wordCount);
return 0;
}
```
### 回答3:
一种基础的算法是利用循环遍历文本,遇到空格或者换行符时将单词计数加一。下面是使用这种算法的C语言代码:
```c
#include <stdio.h>
int countWords(char text[]) {
int count = 0;
int i = 0;
while (text[i] != '\0') {
if (text[i] == ' ' || text[i] == '\n') {
count++;
}
i++;
}
return count + 1; // 最后一个单词后没有空格或者换行符,所以需要加1
}
int main() {
char text[100];
printf("请输入一行文本:");
fgets(text, sizeof(text), stdin);
int wordCount = countWords(text);
printf("单词个数为:%d\n", wordCount);
return 0;
}
```
另一种算法是使用字符串strtok函数将文本分割成单词,并统计分割后的单词个数。下面是使用这种算法的C语言代码:
```c
#include <stdio.h>
#include <string.h>
int countWords(char text[]) {
int count = 0;
char delimiters[] = " \n\t"; // 定义分隔符
char* word = strtok(text, delimiters); // 分割第一个单词
while (word != NULL) {
count++;
word = strtok(NULL, delimiters); // 分割下一个单词
}
return count;
}
int main() {
char text[100];
printf("请输入一行文本:");
fgets(text, sizeof(text), stdin);
int wordCount = countWords(text);
printf("单词个数为:%d\n", wordCount);
return 0;
}
```
以上就是使用C语言实现两种算法统计一行文本的单词个数的代码示例。
阅读全文
相关推荐
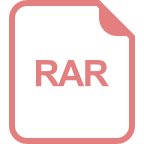
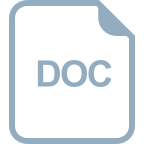
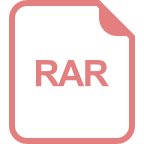
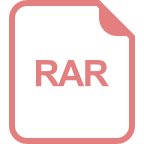
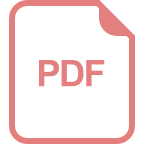
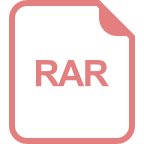
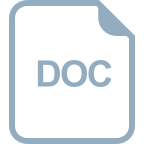
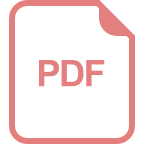
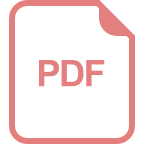
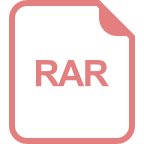
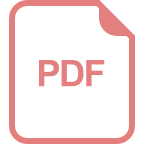
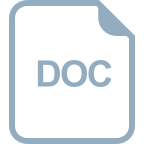