C语言中用两种算法统计一行文本的单词个数,并展示代码
时间: 2023-09-11 12:04:45 浏览: 152
### 回答1:
在C语言中,使用计数法和子字符串法可以统计一行文本的单词个数。计数法的代码如下:int countWords(char *str)
{
int state = 0;
int wc = 0; // word count
// Scan all characters one by one
while (*str)
{
// If next character is a separator, set the state as zero
if (*str == ' ' || *str == '\n' || *str == '\t')
state = 0;
// If next character is not a word separator and state is zero,
// then set the state as 1 and increment word count
else if (state == 0)
{
state = 1;
++wc;
}
// Move to next character
++str;
}
return wc;
} 子字符串法的代码如下:int countWords(char *str)
{
int count = 0;
// A word is separated by space
for (int i = 0; i < strlen(str); i++)
if (str[i] == ' ')
count++;
// Last word is always there
return count + 1;
}
### 回答2:
在C语言中,可以使用两种算法来统计一行文本中的单词个数。
算法一:遍历法
算法思路:
1. 初始化一个计数器变量wordCount为0,表示单词的个数。
2. 遍历文本中的每个字符,从第一个字符开始逐个判断。
3. 如果当前字符是空格、制表符或者换行符,则表示一个单词的结束,将wordCount加1。
4. 继续遍历下一个字符,直到整个文本都被遍历完毕。
5. 返回wordCount。
代码如下所示:
```c
#include <stdio.h>
int countWords(char* text) {
int wordCount = 0;
for (int i = 0; text[i] != '\0'; i++) {
if (text[i] == ' ' || text[i] == '\t' || text[i] == '\n') {
wordCount++;
}
}
return wordCount;
}
int main() {
char text[] = "Hello world. This is a sample text.";
int words = countWords(text);
printf("The number of words in the text is: %d\n", words);
return 0;
}
```
算法二:分割法
算法思路:
1. 初始化一个计数器变量wordCount为0,表示单词的个数。
2. 使用字符串strtok函数将文本根据空格、制表符或者换行符分割为多个子字符串。
3. 遍历每个子字符串,如果该字符串不为空,则表示一个单词,将wordCount加1。
4. 继续寻找下一个子字符串,直到整个文本都被遍历完毕。
5. 返回wordCount。
代码如下所示:
```c
#include <stdio.h>
#include <string.h>
int countWords(char* text) {
int wordCount = 0;
char* word = strtok(text, " \t\n");
while (word != NULL) {
wordCount++;
word = strtok(NULL, " \t\n");
}
return wordCount;
}
int main() {
char text[] = "Hello world. This is a sample text.";
int words = countWords(text);
printf("The number of words in the text is: %d\n", words);
return 0;
}
```
以上就是使用两种算法统计一行文本的单词个数的C语言代码。
### 回答3:
一种算法是使用循环遍历文本字符,通过判断字符是否为空格来计算单词个数。
```c
#include <stdio.h>
int countWords(char *text) {
int count = 0;
int i = 0;
while (text[i] != '\0') {
// 当遇到空格时,说明一个单词结束
if (text[i] == ' ') {
count++;
}
i++;
}
// 针对最后一个单词的情况,如果最后一个字符不是空格,则需要额外计算一个单词
if (text[i - 1] != ' ') {
count++;
}
return count;
}
int main() {
char text[] = "Hello world! This is a test.";
int wordCount = countWords(text);
printf("单词个数:%d\n", wordCount);
return 0;
}
```
另一种算法是使用 strtok 函数将文本分割成单词。
```c
#include <stdio.h>
#include <string.h>
int countWords(char *text) {
int count = 0;
char *word;
// 使用 strtok 分隔字符串
word = strtok(text, " ");
while (word != NULL) {
count++;
word = strtok(NULL, " ");
}
return count;
}
int main() {
char text[] = "Hello world! This is a test.";
int wordCount = countWords(text);
printf("单词个数:%d\n", wordCount);
return 0;
}
```
以上两种算法都可以用来统计一行文本的单词个数,具体使用哪种算法取决于需求和使用场景。
阅读全文
相关推荐
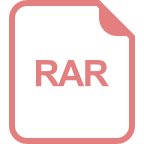
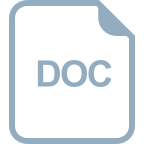
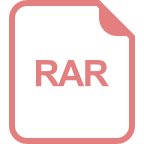
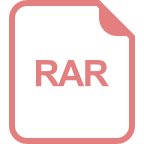
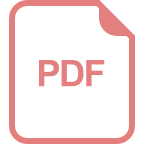
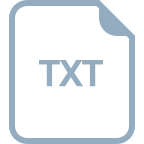
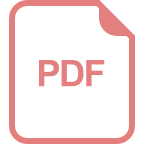
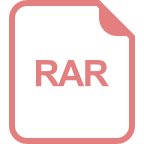
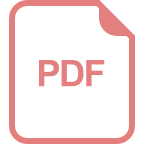
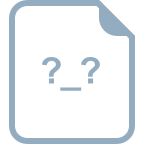
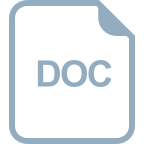