用c语言编写一个程序,描述在 1 到 500 之间随机生成 50 个数字的过程(但不应重复数字),并使用生成的数字生成平衡的探索树
时间: 2024-02-23 22:02:44 浏览: 31
下面是一个用C语言编写的程序,可以生成50个不重复的1到500之间的随机数,并将它们插入到一个平衡的探索树中。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
//定义探索树结构
typedef struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
//生成一个范围在[min, max]之间的随机数
int randInt(int min, int max) {
return rand() % (max - min + 1) + min;
}
//生成一个随机的不重复数字数组
int* genRandomArray(int n, int min, int max) {
int* arr = (int*)malloc(sizeof(int) * n);
int i, j, t;
for (i = 0; i < n; i++) {
arr[i] = randInt(min, max);
for (j = 0; j < i; j++) {
if (arr[j] == arr[i]) {
i--;
break;
}
}
}
return arr;
}
//插入一个结点到探索树中
TreeNode* insert(TreeNode* root, int data) {
if (root == NULL) {
root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = data;
root->left = NULL;
root->right = NULL;
} else if (data < root->data) {
root->left = insert(root->left, data);
} else if (data > root->data) {
root->right = insert(root->right, data);
}
return root;
}
//构建一个平衡的探索树
TreeNode* buildBalancedSearchTree(int* arr, int n) {
TreeNode* root = NULL;
int mid = n / 2;
root = insert(root, arr[mid]);
for (int i = 0; i < mid; i++) {
insert(root, arr[i]);
}
for (int i = mid + 1; i < n; i++) {
insert(root, arr[i]);
}
return root;
}
//中序遍历探索树
void inorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
inorderTraversal(root->left);
printf("%d ", root->data);
inorderTraversal(root->right);
}
int main() {
srand(time(NULL)); //初始化随机数发生器
int* arr = genRandomArray(50, 1, 500);
TreeNode* root = buildBalancedSearchTree(arr, 50);
inorderTraversal(root);
return 0;
}
```
这个程序首先生成一个随机的不重复数字数组,然后将这些数字插入到一个平衡的探索树中。最后,它会对这个探索树进行中序遍历,并输出树中的所有数字。
注意,这个程序中使用了递归来插入和遍历探索树,如果数据量很大,可能会导致栈溢出,需要使用循环或非递归的方法来实现。
相关推荐
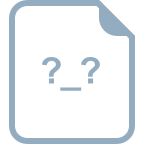
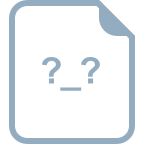














