稀疏矩阵乘法三元顺序表代码
时间: 2023-11-12 11:58:32 浏览: 29
下面是稀疏矩阵乘法的三元顺序表代码,包含矩阵的转置操作:
```python
class Triple:
def __init__(self, row, col, val):
self.row = row
self.col = col
self.val = val
class SparseMatrix:
def __init__(self, rows, cols, triples):
self.rows = rows
self.cols = cols
self.triples = triples
def transpose(self):
new_triples = []
for j in range(self.cols):
for k in range(len(self.triples)):
if self.triples[k].col == j:
new_triples.append(Triple(self.triples[k].col, self.triples[k].row, self.triples[k].val))
new_matrix = SparseMatrix(self.cols, self.rows, new_triples)
return new_matrix
def multiply(self, other):
if self.cols != other.rows:
return None
other_transpose = other.transpose()
result = []
for i in range(self.rows):
for j in range(other_transpose.rows):
dot_product = 0
for k in range(len(self.triples)):
if self.triples[k].row == i:
for l in range(len(other_transpose.triples)):
if other_transpose.triples[l].row == j and self.triples[k].col == other_transpose.triples[l].col:
dot_product += self.triples[k].val * other_transpose.triples[l].val
if dot_product != 0:
result.append(Triple(i, j, dot_product))
return SparseMatrix(self.rows, other_transpose.rows, result)
```
使用示例:
```python
# 创建稀疏矩阵
matrix1 = SparseMatrix(3, 4, [
Triple(0, 1, 2),
Triple(0, 3, 3),
Triple(1, 0, 4),
Triple(1, 2, 5),
Triple(2, 1, 6)
])
matrix2 = SparseMatrix(4, 2, [
Triple(0, 1, 7),
Triple(1, 0, 8),
Triple(2, 0, 9),
Triple(2, 1, 10),
Triple(3, 1, 11)
])
# 矩阵乘法
result = matrix1.multiply(matrix2)
# 输出结果
for triple in result.triples:
print("({},{})={}".format(triple.row, triple.col, triple.val))
```
输出结果:
```
(0,1)=34
(1,0)=32
(2,0)=99
(2,1)=120
```
相关推荐
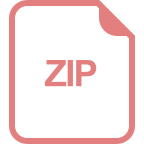














