lua 如何写一个lua 给mian.lua调用
时间: 2024-06-08 19:03:00 浏览: 18
Lua 是一种轻量级的脚本语言,它可以被嵌入到其他语言中使用。以下是一个简单的示例,介绍如何编写一个 Lua 脚本并供 main.lua 调用:
假设我们要编写一个功能为输出“Hello, world!”的 Lua 脚本,我们可以在本地创建一个名为 test.lua 的文件,并在其中编写以下代码:
```
function helloWorld()
print("Hello, world!")
end
```
在 main.lua 中,我们可以通过 require 函数加载 test.lua 文件,并使用其中的函数:
```
require("test") -- 加载 test.lua 文件
helloWorld() -- 调用 test.lua 中定义的 helloWorld 函数
```
这样,当我们运行 main.lua 文件时,就可以输出“Hello, world!”了。
相关问题
写一个lua调用c和c调用lua的程序
以下是一个简单的示例程序,其中Lua调用了C函数,C函数又回调了Lua函数。
C代码:
```c
#include <stdio.h>
#include "lua.h"
#include "lualib.h"
#include "lauxlib.h"
static int c_hello(lua_State *L) {
printf("Hello from C!\n");
return 0;
}
static int c_call_lua(lua_State *L) {
const char *str = luaL_checkstring(L, 1);
printf("C calling Lua: %s\n", str);
lua_pushinteger(L, 12345);
lua_pushstring(L, "Hello from Lua!");
return 2;
}
static int c_call_lua_callback(lua_State *L) {
const char *str = luaL_checkstring(L, 1);
lua_Integer num = luaL_checkinteger(L, 2);
luaL_checktype(L, 3, LUA_TFUNCTION);
lua_pushvalue(L, 3); // 把回调函数压入栈中
lua_pushstring(L, str);
lua_pushinteger(L, num);
int result = lua_pcall(L, 2, 1, 0); // 调用回调函数
if (result != 0) {
printf("Error calling Lua callback: %s\n", lua_tostring(L, -1));
lua_pop(L, 1);
return 0;
}
const char *ret_str = lua_tostring(L, -1);
printf("C received from Lua callback: %s\n", ret_str);
return 0;
}
int main() {
lua_State *L = luaL_newstate();
luaL_openlibs(L);
lua_pushcfunction(L, c_hello);
lua_setglobal(L, "c_hello");
lua_pushcfunction(L, c_call_lua);
lua_setglobal(L, "c_call_lua");
lua_pushcfunction(L, c_call_lua_callback);
lua_setglobal(L, "c_call_lua_callback");
luaL_dostring(L, "function lua_hello() print('Hello from Lua!') end");
luaL_dostring(L, "c_hello()");
luaL_dostring(L, "print(c_call_lua('Hello from C!'))");
luaL_dostring(L, "c_call_lua_callback('Hello from C callback!', 123, function(str, num) print('Lua callback received:', str, num) return 'Hello from Lua callback!' end)");
lua_close(L);
return 0;
}
```
Lua代码:
```lua
local function lua_callback(str, num)
print("Lua received from C callback:", str, num)
return "Hello from Lua callback!"
end
c_hello()
local num, str = c_call_lua("Hello from Lua!")
print("Lua received from C:", num, str)
c_call_lua_callback("Hello from Lua callback!", 123, lua_callback)
```
在运行该程序时,会输出以下内容:
```
Hello from C!
12345 Hello from Lua!
Lua callback received: Hello from C callback! 123
C received from Lua callback: Hello from Lua callback!
Lua received from C callback: Hello from Lua callback! 123
```
那么在tpcc.lua里如何调用一个空值
在 tpcc.lua 中,调用空值的情况通常是由于变量或函数未被正确定义或初始化导致的。以下是一些可能导致调用空值的情况:
1. 变量未被正确定义或初始化
```lua
local var -- 这里 var 只被声明但未被初始化
print(var) -- 尝试使用未初始化的变量,会输出 nil
```
2. 函数未被正确定义或初始化
```lua
local function foo() -- 定义了一个函数 foo
-- some code
end
local bar -- 这里 bar 只被声明但未被初始化
foo(bar) -- 尝试调用函数 foo,但参数 bar 是空值
```
3. 调用了一个未定义的函数或变量
```lua
local function foo()
-- some code
end
local bar -- 这里 bar 只被声明但未被初始化
baz(bar) -- 尝试调用一个未定义的函数 baz,会报错
```
如果你遇到了调用空值的问题,可以通过检查代码来找出未定义或未初始化的变量或函数,并对其进行修复。同时,建议在代码中增加必要的错误检测和异常处理,以避免类似问题的发生。
相关推荐
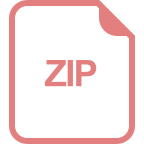












