罗比机器人的遗传算法的py代码
时间: 2024-09-12 12:08:07 浏览: 43
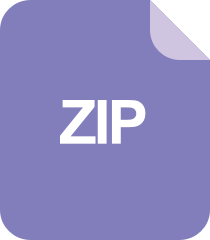
robby-genetic-algorithm:遗传算法创建进化的清洁机器人
遗传算法是模拟自然选择和遗传学机制的搜索优化算法。在编写罗比机器人的遗传算法时,我们通常需要定义种群、适应度函数、选择、交叉(杂交)和变异等基本操作。下面是一个简化的Python代码示例,展示了如何实现一个基本的遗传算法框架:
```python
import random
# 定义罗比机器人的一个简单表示,例如一个由0和1组成的字符串
def create_robot():
return ''.join([str(random.randint(0, 1)) for _ in range(10)])
# 适应度函数,根据罗比机器人的行为定义其适应度
def fitness(robot):
# 这里简单定义,1的数量越多,适应度越高
return sum([int(digit) for digit in robot])
# 选择过程,这里使用轮盘赌选择方法
def select(population):
# 计算种群中所有个体的适应度总和
total_fitness = sum([fitness(robot) for robot in population])
# 生成一个0到总适应度之间的随机数
target = random.uniform(0, total_fitness)
current = 0
for robot in population:
current += fitness(robot)
if current >= target:
return robot
# 交叉过程,这里使用单点交叉
def crossover(parent1, parent2):
# 随机选择一个交叉点
crossover_point = random.randint(1, len(parent1) - 2)
# 交换两个父代的部分基因
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异过程,这里简单地随机改变一个基因
def mutate(robot):
index = random.randint(0, len(robot) - 1)
robot = list(robot)
robot[index] = str(1 - int(robot[index]))
return ''.join(robot)
# 主程序
def genetic_algorithm():
# 创建初始种群
population = [create_robot() for _ in range(50)]
# 进化代数计数器
generation = 0
while generation < 100:
# 计算种群中每个个体的适应度
population = [robot for robot in population if fitness(robot) > 0]
# 如果种群中只剩下一个个体,则停止算法
if len(population) == 1:
break
# 生成下一代
next_generation = []
while len(next_generation) < len(population):
# 选择两个父代
parent1, parent2 = select(population), select(population)
# 交叉产生子代
child1, child2 = crossover(parent1, parent2)
# 变异
child1, child2 = mutate(child1), mutate(child2)
# 将子代替换到下一代种群中
next_generation.extend([child1, child2])
population = next_generation
generation += 1
# 输出最优解
best_robot = max(population, key=fitness)
print("最优解:", best_robot)
# 运行遗传算法
genetic_algorithm()
```
这个代码提供了一个基本的遗传算法的实现框架。请注意,实际应用中罗比机器人的行为和适应度函数可能要复杂得多,且需要根据具体问题进行设计。此外,选择、交叉和变异的具体实现方式也可以根据实际需要进行调整和优化。
阅读全文
相关推荐
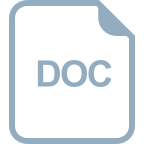


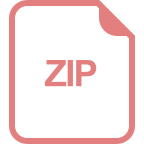
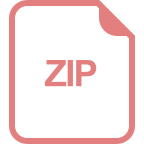
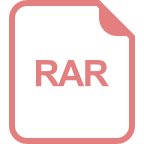
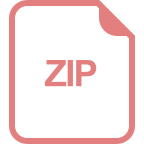
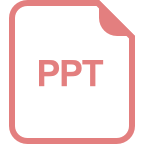
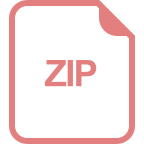
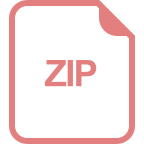
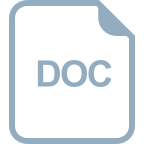
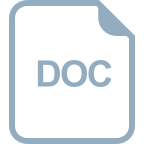

