将大量dat二进制数据文件转成文本文件 python
时间: 2023-10-27 16:07:10 浏览: 53
将dat二进制数据文件转换为文本文件可以使用Python的标准库`numpy`。下面是一个简单的示例程序,将dat二进制文件转换为文本文件:
```python
import numpy as np
# dat二进制文件路径
dat_file = "data.dat"
# 文本文件路径
txt_file = "data.txt"
# 读取dat二进制文件
data = np.fromfile(dat_file, dtype=np.float32)
# 将数据写入文本文件
with open(txt_file, "w") as f_out:
for d in data:
f_out.write(str(d) + "\n")
```
这个程序将读取名为`data.dat`的dat二进制文件,并将其转换为浮点数数组。然后,将数组中的每个浮点数写入名为`data.txt`的文本文件中。请注意,写入文本文件时需要将浮点数转换为字符串,并添加换行符以分隔每个数。
相关问题
python读取二进制文件为字节
Python提供了read()方法来读取二进制文件并将其作为字节返回。read()方法从文件中读取指定数量的字节,并将其存储在一个字节对象中。以下是使用Python读取二进制文件为字节的示例代码:
```python
with open('binary_file.dat', 'rb') as file:
byte_data = file.read()
```
在上面的代码中,我们打开了一个名为 'binary_file.dat' 的二进制文件,并使用 'rb' 模式来表示我们要以二进制模式读取文件。然后,我们使用read()方法从文件中读取所有字节,并将其存储在byte_data变量中。
读取完二进制文件后,我们可以对字节进行进一步的处理,例如将其转换为字符串,或者根据需要解码为其他数据类型(例如整数或浮点数)。
需要注意的是,在使用二进制模式读取文件时,返回的字节对象与文件中的原始字节完全相同,没有进行任何编码或解码操作。因此,对于非文本文件(例如图像或音频文件),将其读取为字节是常见的做法,以便后续处理或分析。
python中文件的类型是 二进制类型有哪些
在 Python 中,文件的类型可以分为文本文件和二进制文件。而二进制文件也可以进一步细分为以下几种类型:
1. 图像文件:如 JPEG、PNG、GIF 等格式的图片文件。
2. 音频文件:如 MP3、WAV、AAC 等格式的音频文件。
3. 视频文件:如 MP4、AVI、MKV 等格式的视频文件。
4. 二进制数据文件:如 DAT、BIN、EXE 等格式的二进制数据文件。
5. 压缩文件:如 ZIP、RAR、7Z 等格式的压缩文件。
当然,以上只是常见的二进制文件类型之一,实际上还有很多其他类型的二进制文件,如数据库文件、字体文件、可执行文件等等。
相关推荐
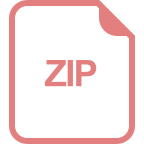
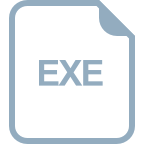












