算术表达式求值。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。不限制方法,你可以用“原始”的多遍扫描字符串,也可以用先进一点的栈(本课程前面接触的栈都是操作系统提供给程序以实现局部变量定义和函数调用的,现在你需要自己创建并使用栈了)。为便于集中精力在功能方面,可以不用检查用户输入的合法性。
时间: 2024-02-01 20:13:57 浏览: 35
本题可以使用栈来实现算术表达式求值。具体做法如下:
1. 创建两个栈,一个用来存储操作数,一个用来存储运算符。
2. 遍历算术表达式,对于每一个字符,进行如下处理:
a. 如果是数字,将其转换为整型,并压入操作数栈。
b. 如果是左括号,将其压入运算符栈。
c. 如果是右括号,弹出运算符栈中的元素,直到遇到左括号,将所有弹出的运算符依次作用于操作数栈中的元素。
d. 如果是运算符,将其与运算符栈顶元素进行比较,如果优先级高于栈顶元素,则将其压入运算符栈;否则,将栈顶运算符弹出,作用于操作数栈中的元素,直到当前运算符优先级大于栈顶元素。
3. 当遍历完整个算术表达式后,将运算符栈中的所有运算符依次弹出,并作用于操作数栈中的元素。
4. 最终,操作数栈中只剩下一个元素,即为算术表达式的结果。
下面是对应的代码实现:
```c++
#include <iostream>
#include <stack>
#include <cstring>
using namespace std;
int priority(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
double calculate(double a, double b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return 0;
}
}
double deal_exp(char *exp) {
stack<double> operands;
stack<char> operators;
int n = strlen(exp);
for (int i = 0; i < n; i++) {
if (isdigit(exp[i]) || (exp[i] == '-' && (i == 0 || !isdigit(exp[i - 1])))) { // 处理数字
int j = i;
while (j < n && (isdigit(exp[j]) || exp[j] == '-')) {
j++;
}
string num_str(exp + i, exp + j);
double num = stod(num_str);
operands.push(num);
i = j - 1;
} else if (exp[i] == '(') { // 处理左括号
operators.push(exp[i]);
} else if (exp[i] == ')') { // 处理右括号
while (!operators.empty() && operators.top() != '(') {
char op = operators.top();
operators.pop();
double b = operands.top();
operands.pop();
double a = operands.top();
operands.pop();
operands.push(calculate(a, b, op));
}
operators.pop(); // 弹出左括号
} else if (exp[i] == '+' || exp[i] == '-' || exp[i] == '*' || exp[i] == '/') { // 处理运算符
while (!operators.empty() && operators.top() != '(' && priority(exp[i]) <= priority(operators.top())) {
char op = operators.top();
operators.pop();
double b = operands.top();
operands.pop();
double a = operands.top();
operands.pop();
operands.push(calculate(a, b, op));
}
operators.push(exp[i]);
}
}
while (!operators.empty()) { // 处理剩余的运算符
char op = operators.top();
operators.pop();
double b = operands.top();
operands.pop();
double a = operands.top();
operands.pop();
operands.push(calculate(a, b, op));
}
return operands.top();
}
int main() {
char exp[1000];
cin >> exp;
double result = deal_exp(exp);
cout << result << endl;
return 0;
}
```
相关推荐
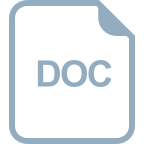





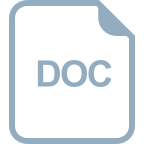
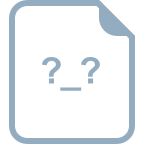
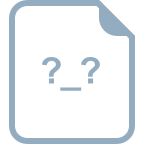
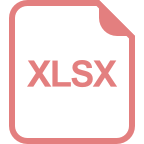
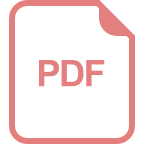
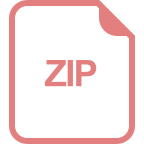