unity物体按抛物线运动代码
时间: 2023-10-08 12:14:52 浏览: 68
以下是Unity中实现物体按抛物线运动的代码示例:
```csharp
public class ParabolicMotion : MonoBehaviour
{
// 起始点、终止点和高度
public Transform startPoint;
public Transform endPoint;
public float height;
// 运动时间
public float timeToReachTarget = 1f;
private bool isMoving = false;
private float timer = 0f;
private Vector3 startPosition;
private Vector3 endPosition;
void Start()
{
// 获取起始点和终止点的位置
startPosition = startPoint.position;
endPosition = endPoint.position;
}
void Update()
{
if (isMoving)
{
// 计算当前时间与总时间的比例
float t = timer / timeToReachTarget;
// 计算当前位置
Vector3 currentPos = ParabolicInterpolation(startPosition, endPosition, height, t);
// 更新物体位置
transform.position = currentPos;
// 更新计时器
timer += Time.deltaTime;
// 判断是否到达终点
if (timer >= timeToReachTarget)
{
isMoving = false;
timer = 0f;
}
}
}
public void StartMotion()
{
isMoving = true;
}
// 抛物线插值函数
private Vector3 ParabolicInterpolation(Vector3 start, Vector3 end, float height, float t)
{
Vector3 result = Vector3.zero;
// 计算抛物线顶点位置
Vector3 vertex = new Vector3((start.x + end.x) / 2f, height, (start.z + end.z) / 2f);
// 计算当前位置
result.x = Mathf.Lerp(start.x, end.x, t);
result.y = Mathf.Lerp(start.y, end.y, t) + Mathf.Sin(t * Mathf.PI) * height;
result.z = Mathf.Lerp(start.z, end.z, t);
return result;
}
}
```
在这个示例中,我们定义了起始点、终止点和高度等参数,然后在Update函数中计算当前位置,利用抛物线插值函数实现物体按抛物线运动。我们还通过StartMotion函数来启动运动,可以通过其他事件来触发该函数。
相关推荐
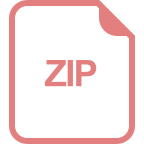
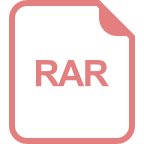
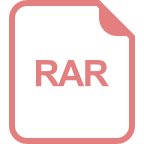
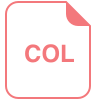













