用python中tensorflow框架在cifar数据集上验证Googlenet神经网络性能,要求最后输出一个最佳epoch的准确率
时间: 2024-02-15 21:01:22 浏览: 21
好的,我可以回答你的问题。首先,需要安装TensorFlow和Keras库。然后,可以使用以下代码来加载CIFAR10数据集:
```python
from keras.datasets import cifar10
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
```
接下来,需要对数据进行预处理,包括归一化和独热编码:
```python
from keras.utils import np_utils
# Normalize the inputs from 0-255 to between 0 and 1
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
# One-hot encode the targets
num_classes = 10
y_train = np_utils.to_categorical(y_train, num_classes)
y_test = np_utils.to_categorical(y_test, num_classes)
```
然后,可以定义GoogLeNet模型:
```python
from keras.models import Model
from keras.layers import Input, concatenate, Dense, Dropout, Flatten, Conv2D, MaxPooling2D, AveragePooling2D, GlobalAveragePooling2D
def inception_module(x, filters):
(branch1, branch2, branch3, branch4) = filters
# 1x1 convolution branch
conv1 = Conv2D(branch1[0], (1, 1), padding='same', activation='relu')(x)
# 1x1 convolution followed by 3x3 convolution branch
conv2 = Conv2D(branch2[0], (1, 1), padding='same', activation='relu')(x)
conv2 = Conv2D(branch2[1], (3, 3), padding='same', activation='relu')(conv2)
# 1x1 convolution followed by 5x5 convolution branch
conv3 = Conv2D(branch3[0], (1, 1), padding='same', activation='relu')(x)
conv3 = Conv2D(branch3[1], (5, 5), padding='same', activation='relu')(conv3)
# 3x3 max pooling followed by 1x1 convolution branch
pool4 = MaxPooling2D((3, 3), strides=(1, 1), padding='same')(x)
conv4 = Conv2D(branch4[0], (1, 1), padding='same', activation='relu')(pool4)
# concatenate the branches
outputs = concatenate([conv1, conv2, conv3, conv4], axis=-1)
return outputs
input = Input(shape=(32, 32, 3))
x = Conv2D(64, (7, 7), strides=(2, 2), padding='same', activation='relu')(input)
x = MaxPooling2D((3, 3), strides=(2, 2), padding='same')(x)
x = Conv2D(64, (1, 1), padding='same', activation='relu')(x)
x = Conv2D(192, (3, 3), padding='same', activation='relu')(x)
x = MaxPooling2D((3, 3), strides=(2, 2), padding='same')(x)
x = inception_module(x, filters=[(64,), (96, 128), (16, 32), (32,)]) # Inception 3a
x = inception_module(x, filters=[(128,), (128, 192), (32, 96), (64,)]) # Inception 3b
x = MaxPooling2D((3, 3), strides=(2, 2), padding='same')(x)
x = inception_module(x, filters=[(192,), (96, 208), (16, 48), (64,)]) # Inception 4a
x = inception_module(x, filters=[(160,), (112, 224), (24, 64), (64,)]) # Inception 4b
x = inception_module(x, filters=[(128,), (128, 256), (24, 64), (64,)]) # Inception 4c
x = inception_module(x, filters=[(112,), (144, 288), (32, 64), (64,)]) # Inception 4d
x = inception_module(x, filters=[(256,), (160, 320), (32, 128), (128,)]) # Inception 4e
x = MaxPooling2D((3, 3), strides=(2, 2), padding='same')(x)
x = inception_module(x, filters=[(256,), (160, 320), (32, 128), (128,)]) # Inception 5a
x = inception_module(x, filters=[(384,), (192, 384), (48, 128), (128,)]) # Inception 5b
x = GlobalAveragePooling2D()(x)
x = Dropout(0.4)(x)
output = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=input, outputs=output)
```
最后,可以编译和训练模型:
```python
from keras.callbacks import ModelCheckpoint
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# Save the model with the best validation accuracy
checkpoint = ModelCheckpoint('best_model.h5', monitor='val_accuracy', save_best_only=True, verbose=1)
# Train the model
history = model.fit(x_train, y_train, validation_data=(x_test, y_test), epochs=50, batch_size=128, callbacks=[checkpoint], verbose=2)
# Load the best model and evaluate it on the test data
model.load_weights('best_model.h5')
score = model.evaluate(x_test, y_test, verbose=0)
print('Best Epoch Test Accuracy:', score[1])
```
在训练过程中,使用ModelCheckpoint回调函数来保存具有最佳验证准确率的模型。最后,加载最佳模型并在测试数据上评估它的性能,输出最佳epoch的准确率。
相关推荐
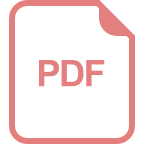
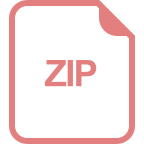
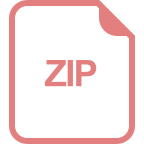














