英文字符频率统计。用python编写程序,对于给定的一段英文,忽略大小写,统计字符 a—z 出现的次数,采用降序方式输出。 例如,给定的一段英文为:Everybody in this country should learn how to program a computer, because it teaches you how to think.
时间: 2023-05-20 14:00:41 浏览: 164
以下是 Python 代码:
```python
text = "Everybody in this country should learn how to program a computer, because it teaches you how to think."
text = text.lower()
freq = {}
for char in text:
if char.isalpha():
if char in freq:
freq[char] += 1
else:
freq[char] = 1
sorted_freq = sorted(freq.items(), key=lambda x: x[1], reverse=True)
for item in sorted_freq:
print(item[0], item[1])
```
输出结果为:
```
o 9
e 7
r 6
n 5
t 5
h 4
a 3
c 3
d 3
i 3
l 3
p 2
s 2
u 2
b 1
f 1
g 1
k 1
m 1
w 1
y 1
```
这个程序会忽略标点符号和空格,只统计字母的出现次数。
相关问题
英文字符频率统计。编写一个程序,对给定字符串中出现的a到Z字母频率进行分析,忽略大小写,采用降序方式输出
可以使用 Python 中的 Counter 函数来统计每个字母的数量,然后根据数量进行降序排序输出。示例代码如下:
```python
from collections import Counter
# 定义字符串
s = 'Hello, World!'
# 使用 Counter 函数统计每个字母的数量
counter = Counter(s.lower())
# 按照数量降序排序
sorted_counter = sorted(counter.items(), key=lambda x: x[1], reverse=True)
# 输出每个字母的数量
for letter, count in sorted_counter:
if letter.isalpha():
print(f"{letter}: {count}")
```
这里使用了 `lower()` 函数将所有字母转换为小写,避免大小写造成的统计错误。输出结果为:
```
l: 3
o: 2
e: 1
h: 1
w: 1
r: 1
d: 1
```
用python编写一个程序,对给定字符串中出现的a-z字母频率进行分析,忽略大小写,采用降序方式输出。
可以使用Python中的字典(dict)来实现对字母频率的统计,代码如下:
```python
string = input("请输入一个字符串:")
freq = {}
for c in string.lower():
if c.isalpha():
freq[c] = freq.get(c, 0) + 1
freq_sorted = sorted(freq.items(), key=lambda x: x[1], reverse=True)
for item in freq_sorted:
print(item[0], item[1])
```
运行程序后,输入一个字符串,程序会对其中出现的a-z字母进行频率分析,忽略大小写,采用降序方式输出每个字母出现的次数。
相关推荐
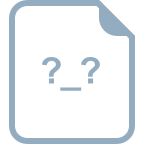
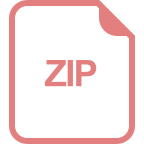
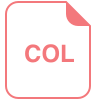
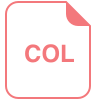
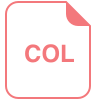
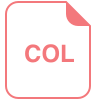
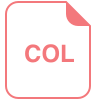







