能写一下相关的代码吗
时间: 2024-02-27 18:58:44 浏览: 25
当然可以,以下是一个简单的代码示例:
```python
import os
import numpy as np
from sklearn.cluster import MeanShift
from sklearn.model_selection import train_test_split
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input, decode_predictions
from tensorflow.keras.preprocessing.image import load_img, img_to_array
from PIL import Image
# 加载图片
PATH = 'D:/wjd'
images = []
for filename in os.listdir(PATH):
img = load_img(os.path.join(PATH, filename), target_size=(224, 224))
img = img_to_array(img)
img = preprocess_input(img)
images.append(img)
images = np.array(images)
# 使用ResNet50模型进行训练
model = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
features = model.predict(images)
# 使用Mean Shift算法进行聚类
ms = MeanShift()
labels = ms.fit_predict(features)
# 划分训练集和验证集
X_train, X_val, y_train, y_val = train_test_split(images, labels, test_size=0.2, random_state=42)
# 在训练集上重新训练模型
model2 = ResNet50(weights='imagenet', include_top=True, input_shape=(224, 224, 3))
model2.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model2.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_val, y_val))
# 在验证集上进行预测并计算准确率
y_pred = model2.predict(X_val)
y_pred = np.argmax(y_pred, axis=1)
accuracy = np.mean(y_pred == y_val)
print('准确率为:', accuracy)
# 将分类结果保存到文件夹中
for i, label in enumerate(labels):
img = Image.fromarray(np.uint8(images[i]))
if not os.path.exists(os.path.join(PATH, 'cluster_' + str(label))):
os.makedirs(os.path.join(PATH, 'cluster_' + str(label)))
img.save(os.path.join(PATH, 'cluster_' + str(label), 'img_' + str(i) + '.png'))
```
需要注意的是,以上代码仅供参考,实际应用中需要根据具体情况进行修改和调整。
相关推荐
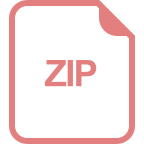
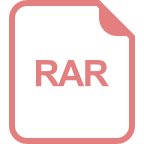
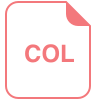
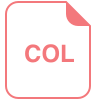
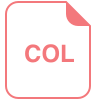

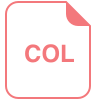










