vue3语法使用canvas或者svg,制作一个宽100px,高50px的心电图动态效果,重复播放
时间: 2023-09-02 10:14:52 浏览: 71
好的,我会用Vue3语法使用canvas制作一个宽100px,高50px的心电图动态效果,并且让它重复播放。
首先需要在Vue3组件中引入canvas元素,然后设置宽高为100px和50px,代码如下:
```html
<template>
<canvas ref="myCanvas" width="100" height="50"></canvas>
</template>
```
接下来,我们需要在组件的mounted钩子函数中获取canvas元素,并且在canvas上绘制出心电图的波形。代码如下:
```javascript
<script>
export default {
mounted() {
const canvas = this.$refs.myCanvas;
const ctx = canvas.getContext('2d');
// 绘制背景颜色
ctx.fillStyle = '#f5f5f5';
ctx.fillRect(0, 0, canvas.width, canvas.height);
// 设置线条颜色和宽度
ctx.strokeStyle = '#ff0000';
ctx.lineWidth = 2;
// 绘制心电图波形
ctx.beginPath();
ctx.moveTo(0, 25);
for (let i = 0; i < 100; i++) {
ctx.lineTo(i * 2, 25 + Math.sin(i * 0.1) * 20);
}
ctx.stroke();
}
};
</script>
```
上面的代码中,我们先绘制了背景颜色,然后设置了线条的颜色和宽度。接着,我们使用moveTo方法将绘图起始点移动到(0,25)的位置,然后使用for循环绘制心电图的波形。最后使用stroke方法将波形绘制出来。
为了让心电图动态播放,我们可以使用setInterval函数定时清除canvas并重新绘制波形。代码如下:
```javascript
<script>
export default {
mounted() {
const canvas = this.$refs.myCanvas;
const ctx = canvas.getContext('2d');
// 绘制背景颜色
ctx.fillStyle = '#f5f5f5';
ctx.fillRect(0, 0, canvas.width, canvas.height);
// 设置线条颜色和宽度
ctx.strokeStyle = '#ff0000';
ctx.lineWidth = 2;
// 绘制心电图波形
const draw = () => {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.moveTo(0, 25);
for (let i = 0; i < 100; i++) {
ctx.lineTo(i * 2, 25 + Math.sin(i * 0.1 + Date.now() * 0.01) * 20);
}
ctx.stroke();
};
setInterval(draw, 30);
}
};
</script>
```
上面的代码中,我们将绘制心电图波形的代码封装成了一个函数draw,并且使用setInterval函数每隔30毫秒调用一次该函数。在绘制波形的时候,我们动态地改变了Math.sin函数中的参数,以达到动态播放的效果。
最后,为了让心电图重复播放,我们可以使用CSS的animation属性来实现。代码如下:
```html
<template>
<canvas ref="myCanvas" width="100" height="50" class="heart-beat"></canvas>
</template>
<style>
.heart-beat {
animation: heart-beat 2s linear infinite;
}
@keyframes heart-beat {
0% {
transform: scale(1);
}
50% {
transform: scale(1.1);
}
100% {
transform: scale(1);
}
}
</style>
```
上面的代码中,我们为canvas元素添加了一个class为heart-beat,并且为该class设置了animation属性。通过设置animation属性,我们可以让心电图动态缩放,从而达到重复播放的效果。
至此,我们使用Vue3语法制作了一个宽100px,高50px的心电图动态效果,并且让它重复播放。
相关推荐
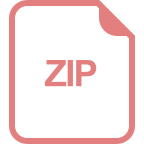
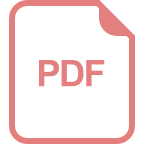














