c#实现低功耗蓝牙ble数据读写功能代码
时间: 2024-03-27 14:37:13 浏览: 164
以下是使用C#实现低功耗蓝牙BLE数据读写功能的示例代码:
```C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
using Windows.Devices.Enumeration;
namespace BleCommunication
{
class Program
{
static void Main(string[] args)
{
var task = MainAsync(args);
task.Wait();
}
static async Task MainAsync(string[] args)
{
// 查询所有蓝牙设备
string selector = BluetoothLEDevice.GetDeviceSelector();
var devices = await DeviceInformation.FindAllAsync(selector);
// 过滤出指定名称的设备
var device = devices.FirstOrDefault(d => d.Name == "My BLE Device");
if (device != null)
{
// 连接设备
var bleDevice = await BluetoothLEDevice.FromIdAsync(device.Id);
// 查询服务
var serviceUuid = Guid.Parse("0000fff0-0000-1000-8000-00805f9b34fb");
var serviceResult = await bleDevice.GetGattServicesForUuidAsync(serviceUuid);
var service = serviceResult.Services.FirstOrDefault();
if (service != null)
{
// 查询特征值
var characteristicUuid = Guid.Parse("0000fff1-0000-1000-8000-00805f9b34fb");
var characteristicResult = await service.GetCharacteristicsForUuidAsync(characteristicUuid);
var characteristic = characteristicResult.Characteristics.FirstOrDefault();
if (characteristic != null)
{
// 订阅特征值通知
var status = await characteristic.WriteClientCharacteristicConfigurationDescriptorAsync(GattClientCharacteristicConfigurationDescriptorValue.Notify);
if (status == GattCommunicationStatus.Success)
{
characteristic.ValueChanged += (sender, args) =>
{
// 处理接收到的数据
var data = args.CharacteristicValue.ToArray();
Console.WriteLine("Received data: {0}", BitConverter.ToString(data));
};
// 发送数据
var dataToSend = new byte[] { 0x01, 0x02, 0x03 };
var writeStatus = await characteristic.WriteValueAsync(dataToSend.AsBuffer());
if (writeStatus == GattCommunicationStatus.Success)
{
Console.WriteLine("Data sent successfully.");
}
else
{
Console.WriteLine("Failed to send data.");
}
}
else
{
Console.WriteLine("Failed to subscribe to notifications.");
}
}
else
{
Console.WriteLine("Characteristic not found.");
}
}
else
{
Console.WriteLine("Service not found.");
}
}
else
{
Console.WriteLine("Device not found.");
}
Console.ReadLine();
}
}
}
```
请注意,此示例仅用于演示BLE通信的基本过程。在实际应用中,您需要根据设备的具体规格和特性来编写更复杂的代码。
阅读全文
相关推荐
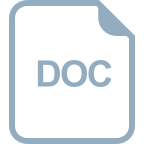
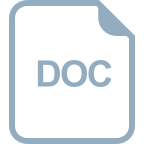
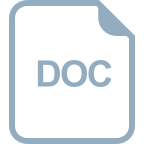

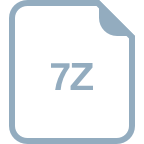
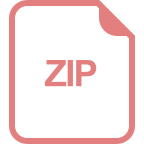
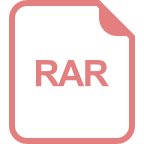

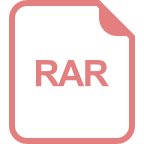
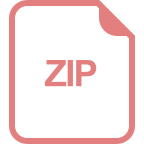
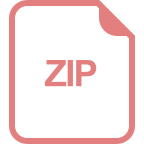
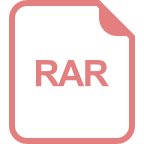
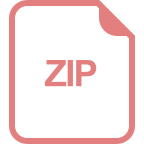
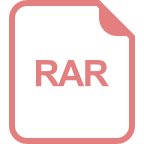
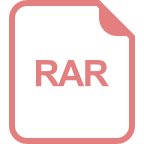
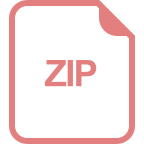
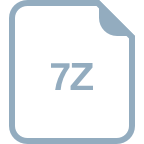
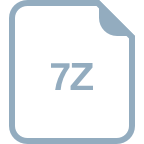