C++点云碰撞测试
时间: 2023-07-04 18:21:09 浏览: 58
点云碰撞测试可以通过计算点云之间的距离和重叠部分来实现。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/kdtree/kdtree_flann.h>
int main()
{
// 创建两个点云
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud1(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud2(new pcl::PointCloud<pcl::PointXYZ>);
// 为点云添加点
cloud1->points.push_back(pcl::PointXYZ(0, 0, 0));
cloud1->points.push_back(pcl::PointXYZ(1, 1, 1));
cloud1->points.push_back(pcl::PointXYZ(2, 2, 2));
cloud2->points.push_back(pcl::PointXYZ(0, 0, 0));
cloud2->points.push_back(pcl::PointXYZ(1, 1, 1));
cloud2->points.push_back(pcl::PointXYZ(3, 3, 3));
// 使用体素滤波器对点云进行下采样
pcl::VoxelGrid<pcl::PointXYZ> sor;
sor.setInputCloud(cloud1);
sor.setLeafSize(0.1f, 0.1f, 0.1f);
sor.filter(*cloud1);
sor.setInputCloud(cloud2);
sor.setLeafSize(0.1f, 0.1f, 0.1f);
sor.filter(*cloud2);
// 使用kdtree对点云进行搜索
pcl::KdTreeFLANN<pcl::PointXYZ> kdtree;
kdtree.setInputCloud(cloud1);
// 计算两个点云之间的距离
float distance = 0;
int count = 0;
for (int i = 0; i < cloud2->points.size(); i++)
{
std::vector<int> indices(1);
std::vector<float> distances(1);
kdtree.nearestKSearch(cloud2->points[i], 1, indices, distances);
if (distances[0] < 0.05f) // 如果距离小于0.05,则认为发生碰撞
{
distance += distances[0];
count++;
}
}
std::cout << "Distance: " << distance << std::endl;
std::cout << "Count: " << count << std::endl;
return 0;
}
```
以上代码中,首先创建了两个点云,然后使用体素滤波器对点云进行下采样,以减少计算量。接着使用kdtree对其中一个点云进行建立索引和搜索,计算两个点云之间的距离,如果距离小于0.05,则认为发生碰撞。
相关推荐
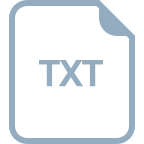














