def resnet18(num_classes=1000, include_top=True): return ResNet(BasicBlock, [2, 2, 2, 2], num_classes=num_classes, include_top=include_top) def resnet34(num_classes=1000, include_top=True): return ResNet(BasicBlock, [3, 4, 6, 3], num_classes=num_classes, include_top=include_top) def resnet50(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 4, 6, 3], num_classes=num_classes, include_top=include_top) def resnet101(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 4, 23, 3], num_classes=num_classes, include_top=include_top) def resnet152(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 8, 36, 3], num_classes=num_classes, include_top=include_top)
时间: 2023-06-26 10:07:51 浏览: 226
这段代码定义了几个函数,用于构建不同深度的 ResNet 模型。ResNet 是一种非常流行的深度学习模型,其主要特点是使用了残差块(Residual Block),可以缓解深度神经网络训练时的梯度消失问题,使得网络可以更深。这里定义了 5 个函数,分别用于构建 ResNet18、ResNet34、ResNet50、ResNet101 和 ResNet152 模型,其中每个函数中的参数都表示不同的深度和宽度。这些模型通常用于图像分类等任务中。
相关问题
为以下每句代码做注释:def resnet18(num_classes=1000, include_top=True): return ResNet(BasicBlock, [2, 2, 2, 2], num_classes=num_classes, include_top=include_top) def resnet34(num_classes=1000, include_top=True): return ResNet(BasicBlock, [3, 4, 6, 3], num_classes=num_classes, include_top=include_top) def resnet50(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 4, 6, 3], num_classes=num_classes, include_top=include_top) def resnet101(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 4, 23, 3], num_classes=num_classes, include_top=include_top) def resnet152(num_classes=1000, include_top=True): return ResNet(Bottleneck, [3, 8, 36, 3], num_classes=num_classes, include_top=include_top)
这段代码定义了5个函数,分别用于构建5个不同深度的ResNet模型,这些函数的参数含义如下:
- num_classes:分类数目,默认为1000
- include_top:是否包含顶层的全连接层,默认为True
这些函数都返回一个ResNet模型,其中BasicBlock和Bottleneck是ResNet中的两种基本块,[2, 2, 2, 2]、[3, 4, 6, 3]、[3, 4, 6, 3]、[3, 4, 23, 3]、[3, 8, 36, 3]分别代表这些ResNet模型所包含的基本块数目。这些函数可以用于构建不同深度的ResNet模型,以适应不同的图像分类任务。
为以下的每句代码做注释:class ResNet(nn.Module): def __init__(self, block, blocks_num, num_classes=1000, include_top=True): super(ResNet, self).__init__() self.include_top = include_top self.in_channel = 64 self.conv1 = nn.Conv2d(3, self.in_channel, kernel_size=7, stride=2, padding=3, bias=False) self.bn1 = nn.BatchNorm2d(self.in_channel) self.relu = nn.ReLU(inplace=True) self.maxpool = nn.MaxPool2d(kernel_size=3, stride=2, padding=1) self.layer1 = self._make_layer(block, 64, blocks_num[0]) self.layer2 = self._make_layer(block, 128, blocks_num[1], stride=2) self.layer3 = self._make_layer(block, 256, blocks_num[2], stride=2) self.layer4 = self._make_layer(block, 512, blocks_num[3], stride=2) if self.include_top: self.avgpool = nn.AdaptiveAvgPool2d((1, 1)) # output size = (1, 1) self.fc = nn.Linear(512 * block.expansion, num_classes) for m in self.modules(): if isinstance(m, nn.Conv2d): nn.init.kaiming_normal_(m.weight, mode='fan_out', nonlinearity='relu') def _make_layer(self, block, channel, block_num, stride=1): downsample = None if stride != 1 or self.in_channel != channel * block.expansion: downsample = nn.Sequential( nn.Conv2d(self.in_channel, channel * block.expansion, kernel_size=1, stride=stride, bias=False), nn.BatchNorm2d(channel * block.expansion)) layers = [] layers.append(block(self.in_channel, channel, downsample=downsample, stride=stride)) self.in_channel = channel * block.expansion for _ in range(1, block_num): layers.append(block(self.in_channel, channel)) return nn.Sequential(*layers) def forward(self, x): x = self.conv1(x) x = self.bn1(x) x = self.relu(x) x = self.maxpool(x) x = self.layer1(x) x = self.layer2(x) x = self.layer3(x) x = self.layer4(x) if self.include_top: x = self.avgpool(x) x = torch.flatten(x, 1) x = self.fc(x) return x
这段代码定义了一个名为 ResNet 的类,继承自 nn.Module 类。ResNet 是一个深度卷积神经网络模型,常用于图像分类任务。
在 __init__ 方法中,首先定义了一些基本参数:
- block:指定 ResNet 中的基本块类型,如 BasicBlock 或 Bottleneck。
- blocks_num:指定每个 stage 中基本块的数量,是一个长度为 4 的列表。
- num_classes:指定模型输出分类数目,默认为 1000。
- include_top:指定是否包含顶层分类器,默认为 True。
接着在 __init__ 中,定义了一些基本组件:
- in_channel:表示当前卷积层的输入通道数,初始化为 64。
- conv1:第一个卷积层,输入 3 通道,输出 self.in_channel 个通道,卷积核大小为 7,步长为 2,padding 为 3。
- bn1:卷积层后面紧跟的批归一化层。
- relu:ReLU 激活函数。
- maxpool:最大池化层,尺寸为 3x3,步长为 2,padding 为 1。
- layer1 - layer4:四个 stage,每个 stage 由多个基本块组成。通过调用 _make_layer 方法生成,该方法会返回一个 Sequential 对象。
- avgpool:全局平均池化层,将特征图转换为 1x1 大小的张量。
- fc:全连接层,将特征向量映射到 num_classes 维空间上。
接下来是 _make_layer 方法,用于生成 ResNet 的每个 stage。该方法包含以下参数:
- block:基本块类型。
- channel:该 stage 中第一个基本块的输出通道数。
- block_num:该 stage 中基本块的数量。
- stride:该 stage 中第一个基本块的步长,默认为 1。
_make_layer 方法首先会根据 stride 和 in_channel 是否等于 channel * block.expansion 构建 downsample 层。如果两者不相等,则 downsample 为一个包含一个卷积层和一个批归一化层的 Sequential 对象。否则,downsample 为 None。
接着,_make_layer 方法通过循环调用 block 方法构建基本块,并将其加入 layers 列表中。其中,第一个基本块的步长由 stride 参数指定,后续基本块步长均为 1。最后,_make_layer 方法返回一个 Sequential 对象,该对象包含所有生成的基本块。
最后是 forward 方法,用于前向传播计算。该方法首先执行一些基本卷积操作,如卷积、批归一化和 ReLU 激活。然后,将特征图 x 依次经过四个 stage,中间可能存在池化和下采样操作。最后,如果 include_top=True,则通过全局平均池化层和全连接层将特征向量映射到 num_classes 维空间上。最终返回分类结果。
阅读全文
相关推荐
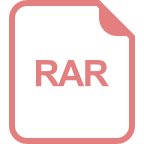

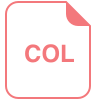
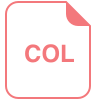
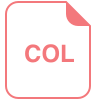
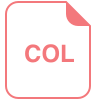
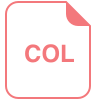
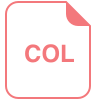
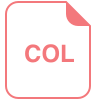
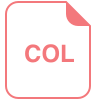
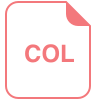
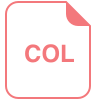
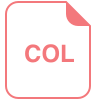
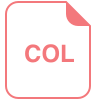
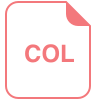