public static BigInteger sqrt(BigInteger n) { BigInteger a = BigInteger.ONE; BigInteger b = n.shiftRight(5).add(BigInteger.valueOf(8)); while (b.compareTo(a) >= 0) { BigInteger mid = a.add(b).shiftRight(1); if (mid.multiply(mid).compareTo(n) > 0) { b = mid.subtract(BigInteger.ONE); } else { a = mid.add(BigInteger.ONE); } } return a.subtract(BigInteger.ONE); }这段逻辑有什么用
时间: 2024-04-27 08:25:30 浏览: 82
这段代码实现了一个用于计算大整数平方根的函数。它使用了二分查找算法来逐步逼近平方根。
具体来说,函数接受一个BigInteger类型的参数n作为输入,然后使用两个BigInteger类型的变量a和b来表示当前的平方根范围。一开始,a被初始化为1,而b则被初始化为n右移5位再加上8,这个值在实践中表现较好。
接下来,函数进入一个while循环,只要b大于等于a,就一直执行。在循环中,函数首先计算中间值mid,即a和b的平均值。然后,函数判断mid的平方是否大于n。如果mid的平方大于n,则说明平方根应该在a和mid之间,因此将b更新为mid-1。否则,平方根应该在mid和b之间,因此将a更新为mid+1。
当b小于a时,循环结束,此时a的值就是n的平方根的整数部分。最后,函数返回a-1,因为实际的平方根可能略小于a。
这个函数的主要用途是在需要处理大整数的情况下计算平方根。由于Java中的原生类型无法表示超出其范围的整数,因此需要使用BigInteger类型来进行计算。而这个函数则提供了一种高效的算法来计算大整数的平方根。
相关问题
import java.math.BigInteger;import java.util.ArrayList;import java.util.List;import java.util.Random;public class PrimitiveRoot { public static int findPrimitiveRoot(int p) { List<Integer> factors = factorize(p - 1); // 计算 p-1 的质因数 Random rand = new Random(); while (true) { int candidate = rand.nextInt(p - 2) + 2; // 随机选取一个 a boolean isPrimitiveRoot = true; for (int factor : factors) { BigInteger exp = BigInteger.valueOf((p - 1) / factor); BigInteger res = BigInteger.valueOf(candidate).modPow(exp, BigInteger.valueOf(p)); if (res.equals(BigInteger.ONE)) { isPrimitiveRoot = false; break; } } if (isPrimitiveRoot) { return candidate; } } } private static List<Integer> factorize(int n) { List<Integer> factors = new ArrayList<>(); for (int i = 2; i <= Math.sqrt(n); i++) { while (n % i == 0) { factors.add(i); n /= i; } } if (n > 1) { factors.add(n); } return factors; } public static void main(String[] args) { int p = 2147483647; int primitiveRoot = findPrimitiveRoot(p); System.out.println("The primitive root of " + p + " is " + primitiveRoot); }}解释代码
这段代码是用Java实现计算一个质数p的原根。具体实现过程如下:
1. 首先调用factorize函数对p-1进行质因数分解,得到p-1的所有质因数。
2. 然后使用随机数选取一个介于2到p-1之间的整数作为候选的原根。
3. 对于p-1的每个质因数,计算它们的指数并将候选原根的对应次幂取模p的结果与1进行比较。
4. 如果有一个质因数的指数使得对应次幂取模p的结果等于1,那么候选原根不是原根,继续随机选取新的候选原根。
5. 如果候选原根对于p-1的每个质因数都不是原根,那么它就是p的原根,函数返回该候选原根。
6. 最后在main函数中调用findPrimitiveRoot函数来计算2147483647的原根,并将结果输出。
总的来说,这段代码实现了对质数的原根的计算,可以用于密码学中的一些算法。
public static BigInteger getFactorCount(BigInteger num) { BigInteger count = new BigInteger("0"); // 计数结果 BigInteger zero = BigInteger.ZERO; // BigInteger内置字段,代表0 BigInteger one = BigInteger.ONE; // BigInteger内置字段,代表1 // 在此完成方法的定义,获取参数num的因子的个数 return count; } }
要获取一个 BigInteger 对象的因子个数,可以将其分解质因数,然后根据质因数的指数计算因子个数。具体步骤如下:
1. 判断 num 是否为质数。如果是,因子个数为 2(1 和 num)。
2. 如果 num 不是质数,将其分解质因数。可以使用一个循环,从 2 开始递增,依次找到 num 的最小质因子,并计算其指数。具体步骤如下:
a. 如果 num 可以被 2 整除,将 2 的指数计数器加 1,然后将 num 除以 2。
b. 如果 num 不可以被 2 整除,从 3 开始,每次增加 2,依次找到 num 的最小奇数质因子,并计算其指数。具体步骤如下:
i. 如果 num 可以被当前质因子整除,将其指数计数器加 1,然后将 num 除以该质因子。
ii. 如果 num 不可以被当前质因子整除,换下一个奇数质因子。
iii. 当质因子的平方大于 num 时,停止循环。
3. 计算因子个数。如果 num 是质数,因子个数为 2;否则,因子个数为各个质因子指数加 1 的乘积。
下面是代码实现:
```
public static BigInteger getFactorCount(BigInteger num) {
BigInteger count = BigInteger.ONE; // 计数结果,初始为1
BigInteger two = BigInteger.valueOf(2); // BigInteger内置静态方法,返回值为2的BigInteger对象
if (num.compareTo(two) < 0) { // 如果num小于2,返回0
return BigInteger.ZERO;
}
BigInteger factor = two; // 当前质因子,初始为2
BigInteger exp = BigInteger.ZERO; // 当前质因子的指数,初始为0
while (num.mod(factor).equals(BigInteger.ZERO)) { // 如果num可以被2整除
exp = exp.add(BigInteger.ONE); // 将2的指数计数器加1
num = num.divide(factor); // 将num除以2
}
if (num.equals(BigInteger.ONE)) { // 如果num已经被分解完毕
return exp.add(BigInteger.ONE); // 返回2的指数加1
}
BigInteger limit = sqrt(num); // 计算最大质因子,即num的平方根
factor = BigInteger.valueOf(3); // 从3开始找奇数质因子
while (factor.compareTo(limit) <= 0) { // 如果质因子小于等于最大质因子
exp = BigInteger.ZERO; // 将指数计数器重置为0
while (num.mod(factor).equals(BigInteger.ZERO)) { // 如果num可以被当前质因子整除
exp = exp.add(BigInteger.ONE); // 将当前质因子的指数计数器加1
num = num.divide(factor); // 将num除以当前质因子
}
if (!exp.equals(BigInteger.ZERO)) { // 如果num可以被当前质因子整除
count = count.multiply(exp.add(BigInteger.ONE)); // 更新计数结果
}
factor = factor.add(two); // 下一个奇数质因子
}
if (!num.equals(BigInteger.ONE)) { // 如果num是一个大于sqrt(num)的质数
count = count.multiply(BigInteger.TWO); // 更新计数结果
}
return count; // 返回计数结果
}
public static BigInteger sqrt(BigInteger num) {
BigInteger sqrt = num.shiftRight(num.bitLength() / 2); // 初始平方根为num的二进制位数的一半
while (sqrt.multiply(sqrt).compareTo(num) > 0) { // 如果平方根的平方大于num
sqrt = sqrt.add(num.divide(sqrt)).divide(BigInteger.TWO); // 使用牛顿迭代法逼近平方根
}
return sqrt; // 返回平方根
}
```
阅读全文
相关推荐
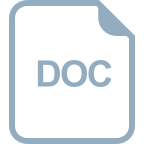
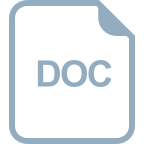
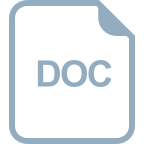

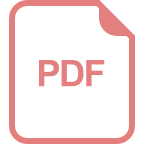
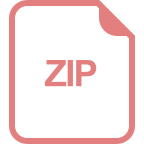
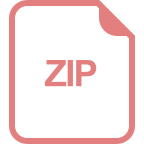
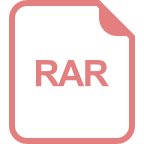
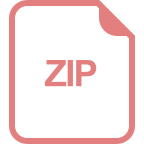
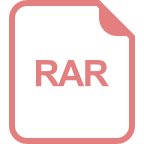
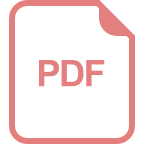
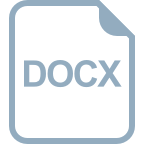





