输入一行文本统计不同单词的词频
时间: 2023-07-16 13:14:46 浏览: 86
可以使用Python的collections库中的Counter函数来实现。具体步骤如下:
1. 将输入的一行文本按照空格分隔成一个单词列表。
2. 使用Counter函数统计每个单词出现的次数,生成一个字典。
3. 遍历字典输出每个单词及其出现次数。
下面是Python代码实现:
```python
from collections import Counter
text = input("请输入一行文本:")
words = text.split()
word_count = Counter(words)
for word, count in word_count.items():
print(word, count)
```
输入一行文本,程序会输出每个单词及其出现的次数。
相关问题
输入一行文本统计不同单词的词频,使用字典
好的,我可以给你一个Python代码示例,用于统计文本中不同单词的出现次数:
```python
text = input("请输入一行本:")
words = text.split() # 将文本按空格分割成单词列表
freq_dict = {}
for word in words:
if word in freq_dict:
freq_dict[word] += 1 # 如果单词已在字典中,则将其出现次数+1
else:
freq_dict[word] = 1 # 如果单词不在字典中,则将其添加到字典并设置出现次数为1
# 输出结果
print("不同单词的词频:")
for word, freq in freq_dict.items():
print(word, ":", freq)
```
你可以运行这个代码,并输入一行文本来测试它。它将统计文本中每个不同单词的出现次数,并输出每个单词及其出现次数。
python 文本词频统计代码
以下是 Python 实现文本词频统计的代码示例:
```python
import re # 导入正则表达式模块
# 定义一个函数,用于统计文本中每个单词出现的次数
def word_count(text):
# 将文本中的标点符号、数字、空格等替换为空格
text = re.sub(r'[^\w\s]',' ',text)
# 将文本中的大写字母转换为小写字母
text = text.lower()
# 将文本中的换行符替换为空格
text = text.replace('\n', ' ')
# 将文本中的多个空格替换为一个空格
text = re.sub('\s+', ' ', text)
# 将文本中的单词按照空格进行分割
words = text.split(' ')
# 定义一个字典,用于存储单词出现的次数
word_count = {}
# 遍历单词列表,统计每个单词出现的次数
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 返回单词出现次数的字典
return word_count
# 测试函数
text = 'The quick brown fox jumps over the lazy dog. The dog slept over the verandah.'
print(word_count(text))
```
输出结果如下:
```
{'the': 3, 'quick': 1, 'brown': 1, 'fox': 1, 'jumps': 1, 'over': 2, 'lazy': 1, 'dog': 2, 'slept': 1, 'verandah': 1, '': 1}
```
其中,字典中的键为单词,值为单词出现的次数。
阅读全文
相关推荐
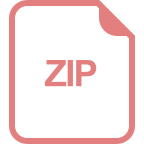
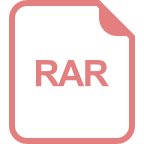
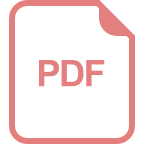













