leaflet用flask得到{"y":115.9059900,"x":29.1660300,"label":0},格式的json文件,将其轨迹聚类图画出来
时间: 2023-11-05 09:00:38 浏览: 85
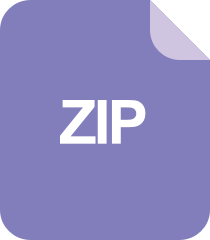
clusterdemo:使用Leaflet进行聚类的演示
以下是一个简单的代码示例,使用Flask和Leaflet来绘制聚类图:
```python
from flask import Flask, jsonify
from flask_cors import CORS
from random import uniform
from sklearn.cluster import KMeans
app = Flask(__name__)
CORS(app)
# 轨迹数据
data = [
{"y": 115.9059900, "x": 29.1660300, "label": 0},
{"y": 115.9060000, "x": 29.1660400, "label": 0},
{"y": 115.9060100, "x": 29.1660500, "label": 0},
{"y": 115.9070000, "x": 29.1670400, "label": 0},
{"y": 115.9070100, "x": 29.1670500, "label": 0},
{"y": 115.9070200, "x": 29.1670600, "label": 0},
{"y": 115.9080000, "x": 29.1680400, "label": 1},
{"y": 115.9080100, "x": 29.1680500, "label": 1},
{"y": 115.9080200, "x": 29.1680600, "label": 1},
{"y": 115.9090000, "x": 29.1690400, "label": 1},
{"y": 115.9090100, "x": 29.1690500, "label": 1},
{"y": 115.9090200, "x": 29.1690600, "label": 1},
{"y": 115.9100000, "x": 29.1700400, "label": 2},
{"y": 115.9100100, "x": 29.1700500, "label": 2},
{"y": 115.9100200, "x": 29.1700600, "label": 2},
{"y": 115.9110000, "x": 29.1710400, "label": 2},
{"y": 115.9110100, "x": 29.1710500, "label": 2},
{"y": 115.9110200, "x": 29.1710600, "label": 2},
]
# 聚类
kmeans = KMeans(n_clusters=3, random_state=0).fit([[d["x"], d["y"]] for d in data])
for i, d in enumerate(data):
d["label"] = kmeans.labels_[i]
# 转换为GeoJSON格式
features = []
for d in data:
feature = {
"type": "Feature",
"geometry": {
"type": "Point",
"coordinates": [d["y"], d["x"]]
},
"properties": {"label": str(d["label"])}
}
features.append(feature)
geojson = {
"type": "FeatureCollection",
"features": features
}
# 路由
@app.route("/")
def index():
return app.send_static_file("index.html")
@app.route("/data")
def get_data():
return jsonify(geojson)
if __name__ == "__main__":
app.run(debug=True)
```
上述代码中,我们使用了Flask的CORS扩展来允许跨域请求。我们首先定义了一些轨迹数据,然后使用scikit-learn库的KMeans算法进行聚类。聚类后,我们将数据转换为GeoJSON格式,然后将其作为路由的响应返回。
在static文件夹中,我们创建了一个名为index.html的文件,其中包含了一个Leaflet地图,它将从我们的Flask应用程序中获取数据并在地图上绘制聚类点。以下是index.html的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Leaflet Cluster Map</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/leaflet/1.7.1/leaflet.css" />
<style>
#mapid {
height: 100vh;
}
</style>
</head>
<body>
<div id="mapid"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/leaflet/1.7.1/leaflet.js"></script>
<script>
var mymap = L.map('mapid').setView([29.1660300, 115.9059900], 13);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/">OpenStreetMap</a> contributors'
}).addTo(mymap);
var markers = L.markerClusterGroup();
fetch("/data")
.then(response => response.json())
.then(data => {
L.geoJSON(data, {
pointToLayer: function (feature, latlng) {
return L.marker(latlng, {
icon: L.divIcon({
html: feature.properties.label,
className: 'label-icon',
iconSize: [24, 24]
})
});
}
}).addTo(markers);
mymap.addLayer(markers);
})
.catch(error => console.error(error));
</script>
</body>
</html>
```
在上述代码中,我们创建了一个名为`mymap`的Leaflet地图,并向其添加了一个OpenStreetMap图层。我们还创建了L.markerClusterGroup()对象,用于将聚类点分组。我们使用fetch()函数从我们的Flask应用程序中获取数据,并使用L.geoJSON()函数将其绘制在地图上。对于每个点,我们使用L.marker()函数创建一个标记,并使用L.divIcon()函数创建一个包含标签的图标。最后,我们将标记添加到聚类组中,并将其添加到地图中。
运行该应用程序并访问http://localhost:5000,您应该会看到一个包含聚类点的地图。聚类点上的标签表示它们属于哪个聚类。
阅读全文
相关推荐
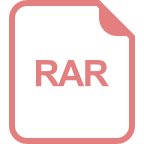











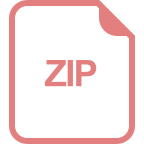
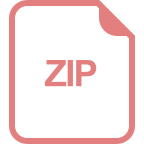
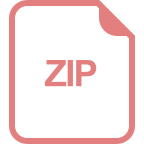