netty实现tcp通信服务端代码
时间: 2023-09-23 17:07:03 浏览: 51
下面是 Netty 实现 TCP 通信服务端的代码示例:
```
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class TcpServer {
private final int port;
public TcpServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.option(ChannelOption.SO_BACKLOG, 1024);
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new TcpServerInitializer());
Channel ch = b.bind(port).sync().channel();
ch.closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port;
if (args.length > 0) {
port = Integer.parseInt(args[0]);
} else {
port = 8080;
}
new TcpServer(port).run();
}
}
```
这段代码使用了 Netty 的 ServerBootstrap 来启动服务端,并使用 NioEventLoopGroup 来处理连接请求和数据传输。
其中 TcpServerInitializer 类是一个自定义的类,它会在服务端的 Channel 中添加处理器,用于处理数据的接收和发送。
相关推荐
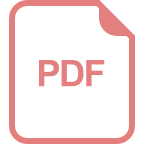
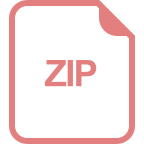
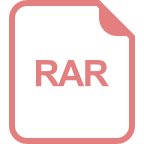
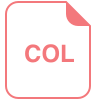
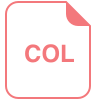
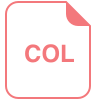
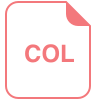
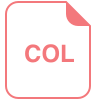









