读取/sys/class/pwm/pwmchip0/polarity的内容demoC语言函数
时间: 2023-09-08 07:16:31 浏览: 46
可以使用以下的C语言函数来读取/sys/class/pwm/pwmchip0/polarity的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BUF 256
int main() {
FILE *fp;
char path[MAX_BUF];
char buf[MAX_BUF];
// 构建文件路径
snprintf(path, sizeof(path), "/sys/class/pwm/pwmchip0/polarity");
// 打开文件
fp = fopen(path, "r");
if (fp == NULL) {
printf("Failed to open file: %s\n", path);
return 1;
}
// 读取文件内容
fgets(buf, sizeof(buf), fp);
// 关闭文件
fclose(fp);
// 输出文件内容
printf("Content of %s: %s", path, buf);
return 0;
}
```
该程序通过构建文件路径,打开并读取文件内容,最后输出文件内容。需要注意的是,该程序只能读取文件内容,不能对文件进行修改。
相关问题
创建一个读取/sys/class/pwm/pwmchip0/polarity的内容demoC语言函数
以下是一个简单的C语言函数,用于读取/sys/class/pwm/pwmchip0/polarity的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_BUF 64
int read_pwm_polarity()
{
int polarity = -1;
char buf[MAX_BUF];
FILE *fp;
fp = fopen("/sys/class/pwm/pwmchip0/polarity", "r");
if (fp == NULL) {
printf("Failed to open polarity file\n");
return polarity;
}
if (fgets(buf, MAX_BUF, fp) != NULL) {
polarity = atoi(buf);
}
fclose(fp);
return polarity;
}
```
这个函数使用标准C库中的fopen()打开/sys/class/pwm/pwmchip0/polarity文件,并使用fgets()函数读取文件中的内容。然后,将读取到的字符串转换为整数并返回。如果无法打开文件,则返回值为-1。请注意,此函数假定文件中包含一个整数值。如果文件格式有所不同,请相应地更改函数。
创建一个读取/sys/class/pwm/pwmchip0/polarity的字符串内容demoC语言函数
你可以使用以下的C语言代码来读取/sys/class/pwm/pwmchip0/polarity文件的内容:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_BUF 256
char* read_pwm_polarity() {
FILE *fp;
char *path = "/sys/class/pwm/pwmchip0/polarity";
char buf[MAX_BUF];
fp = fopen(path, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", path);
return NULL;
}
fgets(buf, MAX_BUF, fp);
fclose(fp);
return buf;
}
int main() {
char *polarity = read_pwm_polarity();
if (polarity != NULL) {
printf("PWM polarity: %s\n", polarity);
free(polarity);
}
return 0;
}
```
这个函数会返回一个指向字符串的指针,该字符串包含了文件的内容。请注意,这个函数并没有做任何的错误检查和处理,你需要根据自己的需要进行补充。
相关推荐
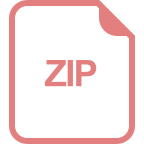
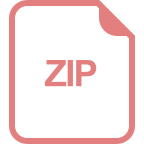
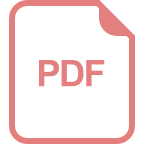












