model small .stack 100h .data msg db 'The string is a palindrome.$' msg1 db 'The string is a palindrome.$' msg2 db 'The string is not a palindrome.$' str1 db 'abccba$' str2 db '1234321$' str3 db 'sdsfds$' .code main proc mov ax, @data mov ds, ax ; 判断字符串1 mov si, 0 mov cx, 0 mov cl, 6 dec cx mov di, cx shr cx, 1 mov bx, 0 cmp cx, bx jle palindrome1 mov bx, 1 outer_loop1: mov al, [str1+si+1] mov ah, [str1+di+1] cmp al, ah jne not_palindrome1 inc si dec di cmp si, di jg palindrome1 inner_loop1: mov al, [str1+si+1] mov ah, [str1+di+1] cmp al, ah jne not_palindrome1 inc si dec di cmp si, di jle inner_loop1 palindrome1: lea dx, msg1 mov ah, 09h int 21h jmp check_next not_palindrome1: lea dx, msg2 mov ah, 09h int 21h check_next: ; 判断字符串2 mov si, 0 mov cx, 0 mov cl, 7 dec cx mov di, cx shr cx, 1 mov bx, 0 cmp cx, bx jle palindrome2 mov bx, 1 outer_loop2: mov al, [str2+si+1] mov ah, [str2+di+1] cmp al, ah jne not_palindrome2 inc si dec di cmp si, di jg palindrome2 inner_loop2: mov al, [str2+si+1] mov ah, [str2+di+1] cmp al, ah jne not_palindrome2 inc si dec di cmp si, di jle inner_loop2 palindrome2: lea dx, msg1 mov ah, 09h int 21h jmp check_next2 not_palindrome2: lea dx, msg2 mov ah, 09h int 21h check_next2: ; 判断字符串3 mov si, 0 mov cx, 0 mov cl, 6 dec cx mov di, cx shr cx, 1 mov bx, 0 cmp cx, bx jle palindrome3 mov bx, 1 outer_loop3: mov al, [str3+si+1] mov ah, [str3+di+1] cmp al, ah jne not_palindrome3 inc si dec di cmp si, di jg palindrome3 inner_loop3: mov al, [str3+si+1] mov ah, [str3+di+1] cmp al, ah jne not_palindrome3 inc si dec di cmp si, di jle inner_loop3 palindrome3: lea dx, msg1 mov ah, 09h int 21h jmp exit_program not_palindrome3: lea dx, msg2 mov ah, 09h int 21h jmp exit_program exit_program: mov ah, 4ch int 21h main endp end main能不能将代码优化用将带判断字符串看为二维数组
时间: 2023-12-09 14:03:48 浏览: 37
可以将代码优化,使用二维数组来存储多个字符串,并使用循环来遍历判断每个字符串是否为回文。以下是优化后的代码:
```
model small
.stack 100h
.data
msg db 'The string is a palindrome.$'
msg1 db 'The string is a palindrome.$'
msg2 db 'The string is not a palindrome.$'
strings db 'abccba$', '1234321$', 'sdsfds$'
num_strings equ 3
str_len equ 7
.code
main proc
mov ax, @data
mov ds, ax
mov si, 0 ; 用si存储字符串的索引
mov cx, 0
mov cl, str_len
dec cx
mov di, cx ; 用di存储字符串的另一个索引(从后往前)
shr cx, 1
mov bx, 0
cmp cx, bx
jle check_next ; 如果字符串长度为0或1,则直接跳到下一个字符串
outer_loop:
mov ah, 0
mov al, num_strings
mul si ; 计算当前字符串在二维数组中的偏移量
add al, strings
mov bx, ax ; 将偏移量存储在bx中
mov si, 0 ; 重置si和di
mov di, str_len - 1
inner_loop:
mov al, [bx+si] ; 从二维数组中读取字符
mov ah, [bx+di]
cmp al, ah
jne not_palindrome
inc si
dec di
cmp si, di
jle inner_loop
palindrome:
lea dx, msg1
mov ah, 09h
int 21h
jmp check_next
not_palindrome:
lea dx, msg2
mov ah, 09h
int 21h
jmp check_next
check_next:
inc si ; 检查下一个字符串
cmp si, num_strings
jl outer_loop
exit_program:
mov ah, 4ch
int 21h
main endp
end main
```
相关推荐
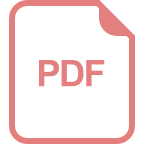








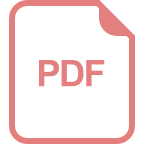
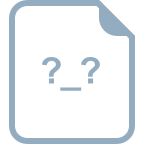
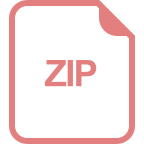
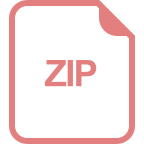
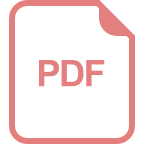
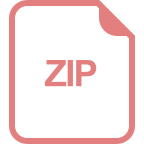