在VS上用c语言写FIR滤波器
时间: 2023-06-27 07:08:02 浏览: 76
下面是一个使用C语言在Visual Studio上实现的FIR滤波器的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define SAMPLE_RATE 44100
#define FILTER_FREQ 1000
double filterCoefficients[101];
void generateFilterCoefficients()
{
int filterLength = 100;
double cutoffFreq = FILTER_FREQ / ((double)SAMPLE_RATE / 2);
for (int n = 0; n <= filterLength; n++) {
if (n == filterLength / 2) {
filterCoefficients[n] = 2 * M_PI * cutoffFreq;
}
else {
filterCoefficients[n] = sin(2 * M_PI * cutoffFreq * (n - filterLength / 2)) / (M_PI * (n - filterLength / 2));
}
filterCoefficients[n] *= 0.54 - 0.46 * cos(2 * M_PI * n / filterLength);
}
}
double filterSample(double sample)
{
static double delayLine[101] = { 0 };
double outputSample = 0;
delayLine[0] = sample;
for (int n = 0; n <= 100; n++) {
outputSample += filterCoefficients[n] * delayLine[n];
}
for (int n = 100; n >= 1; n--) {
delayLine[n] = delayLine[n - 1];
}
return outputSample;
}
int main()
{
generateFilterCoefficients();
// test the filter on a sine wave
double frequency = 500;
double amplitude = 10000;
double phase = 0;
double time = 0;
double sample;
double filteredSample;
for (int i = 0; i < SAMPLE_RATE; i++) {
sample = amplitude * sin(2 * M_PI * frequency * time + phase);
filteredSample = filterSample(sample);
printf("%d, %f, %f\n", i, sample, filteredSample);
time += 1 / (double)SAMPLE_RATE;
}
return 0;
}
```
这个示例代码实现了一个100阶的低通FIR滤波器,截止频率为1000Hz,样本率为44100Hz。`generateFilterCoefficients()`函数生成了FIR滤波器的系数,`filterSample()`函数对每个输入样本进行滤波,并返回输出样本。在`main()`函数中,我们测试了这个滤波器在500Hz正弦波上的效果。最终输出的结果包括样本序号、输入样本值和输出样本值。你可以根据自己的需要修改截止频率、滤波器阶数、样本率等参数。
相关推荐
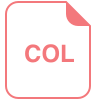
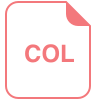
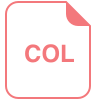
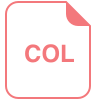
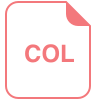









