在tensorflow环境下,用resnet18训练mnist
时间: 2023-06-14 09:04:47 浏览: 82
首先,需要准备好MNIST数据集。可以使用TensorFlow内置的`tf.keras.datasets.mnist`模块来下载和加载数据集:
```python
import tensorflow as tf
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
```
然后,需要对数据进行预处理,将像素值缩放到0到1之间,并将标签转换为独热编码。可以使用`tf.keras.utils.to_categorical`函数实现:
```python
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, num_classes=10)
y_test = tf.keras.utils.to_categorical(y_test, num_classes=10)
```
接下来,需要定义ResNet18模型。可以使用TensorFlow内置的`tf.keras.applications.ResNet18`模块来加载预训练的ResNet18模型,并将其顶层替换为适合MNIST数据集的全连接层:
```python
base_model = tf.keras.applications.ResNet18(
include_top=False, weights='imagenet', input_shape=(28, 28, 1))
x = base_model.output
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = tf.keras.layers.Dense(128, activation='relu')(x)
predictions = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.models.Model(inputs=base_model.input, outputs=predictions)
```
最后,需要编译并训练模型:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
```
完整代码如下:
```python
import tensorflow as tf
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, num_classes=10)
y_test = tf.keras.utils.to_categorical(y_test, num_classes=10)
base_model = tf.keras.applications.ResNet18(
include_top=False, weights='imagenet', input_shape=(28, 28, 1))
x = base_model.output
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = tf.keras.layers.Dense(128, activation='relu')(x)
predictions = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.models.Model(inputs=base_model.input, outputs=predictions)
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
```
相关推荐
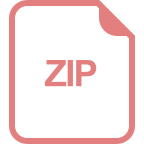
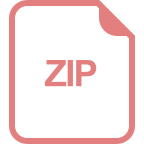
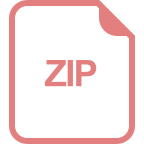














