public static void main(String[] args) { Foo foo = new Foo(); // Thread t1 = new Thread(()->foo.methodA()); Thread t1 = new Thread(foo::methodA); Thread t2 = new Thread(foo::methodB); t1.start(); t2.start(); } } class Foo{ public synchronized void methodA(){ Thread t = Thread.currentThread(); System.out.println(t.getName()+":正在执行A方法..."); try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(t.getName()+":执行A方法完毕"); } public void methodB(){ synchronized(this) { Thread t = Thread.currentThread(); System.out.println(t.getName() + ":正在执行B方法..."); try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(t.getName() + ":执行B方法完毕"); } }
时间: 2023-12-06 21:44:00 浏览: 56
这段代码展示了Java中的synchronized关键字的使用。synchronized关键字可以用来保证多个线程在访问共享资源时的线程安全性,通过对类或对象加锁来实现。
在这个例子中,Foo类的methodA()方法和methodB()方法都是synchronized方法,它们都使用了this对象作为锁。当一个线程执行methodA()方法时,它会获取this对象的锁,其他线程就无法同时执行methodA()方法或methodB()方法,直到该线程释放锁。因此,在本例中,t1和t2线程不能同时执行methodA()方法或methodB()方法,它们必须交替执行。
需要注意的是,在methodB()方法中,使用了synchronized(this)语句块,这与直接将methodB()方法声明为synchronized方法的效果是一样的,但是它的范围更小,只对synchronized(this)中的代码块加锁。这种方式可以避免对整个方法进行加锁,提高了程序的效率。
相关问题
public class Demo03{ public static void main (String[] args){ Foo foo = new Foo(); foo.test(127) }} c;ass Foo{void test(byte a){System.out,println("byte")}}
这段代码存在语法错误,应该是:
```
public class Demo03 {
public static void main(String[] args) {
Foo foo = new Foo();
foo.test((byte) 127);
}
}
class Foo {
void test(byte a) {
System.out.println("byte");
}
}
```
在调用 `foo.test(127)` 时,编译器会将 `127` 当作 `int` 类型来处理,而 `test` 方法的参数类型是 `byte`,因此需要强制类型转换,即 `foo.test((byte) 127)`。此外,代码中的 `class` 关键字拼写错误,应为 `class`。最后,`System.out,println` 方法中的逗号应该改为点号,即 `System.out.println`。
public class X { public static void main(String[] args) { String foo="ABCDE"; foo.substring(3); foo.concat("XYZ"); } }
这段代码定义了一个名为X的公共类。在main方法中,创建了一个名为foo的字符串变量,并将其初始化为"ABCDE"。然后,使用foo.substring(3)方法调用字符串的substring方法,该方法会返回从索引3开始的子字符串,但在此代码中没有接收和使用返回值,所以没有对foo进行修改。
接下来,使用foo.concat("XYZ")方法调用字符串的concat方法,该方法用于将指定的字符串附加到原始字符串的末尾,并返回新的字符串。但同样,代码中没有接收和使用返回值,所以对foo也没有进行修改。
因此,这段代码最终不会改变foo的值。
相关推荐
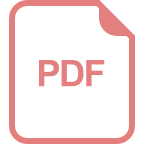
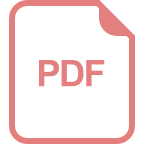
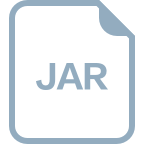











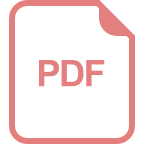
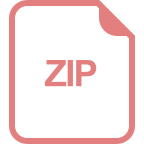