用java编写一个完整的拼图游戏界面
时间: 2024-02-25 14:58:40 浏览: 16
好的,以下是一个用Java Swing编写的拼图游戏界面的示例代码,仅供参考。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class PuzzleGame extends JFrame implements ActionListener {
private JPanel mainPanel;
private JPanel puzzlePanel;
private JLabel timeLabel;
private JLabel stepLabel;
private JButton resetButton;
private JButton quitButton;
private Timer timer;
private int timeCount;
private int stepCount;
private List<JButton> puzzleButtonList;
private final int ROWS = 3;
private final int COLS = 3;
private final int BUTTON_SIZE = 120;
private final int GAP_SIZE = 10;
public PuzzleGame() {
setTitle("拼图游戏");
setSize(400, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
initUI();
initGame();
setVisible(true);
}
private void initUI() {
mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
puzzlePanel = new JPanel();
puzzlePanel.setLayout(new GridLayout(ROWS, COLS, GAP_SIZE, GAP_SIZE));
puzzlePanel.setPreferredSize(new Dimension(BUTTON_SIZE * COLS + GAP_SIZE * (COLS + 1),
BUTTON_SIZE * ROWS + GAP_SIZE * (ROWS + 1)));
timeLabel = new JLabel("0秒");
stepLabel = new JLabel("步数:0步");
resetButton = new JButton("重新开始");
resetButton.addActionListener(this);
quitButton = new JButton("退出游戏");
quitButton.addActionListener(this);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new FlowLayout());
bottomPanel.add(timeLabel);
bottomPanel.add(stepLabel);
bottomPanel.add(resetButton);
bottomPanel.add(quitButton);
mainPanel.add(puzzlePanel, BorderLayout.CENTER);
mainPanel.add(bottomPanel, BorderLayout.SOUTH);
add(mainPanel);
}
private void initGame() {
timeCount = 0;
stepCount = 0;
timeLabel.setText("0秒");
stepLabel.setText("步数:0步");
puzzleButtonList = new ArrayList<>();
for (int i = 0; i < ROWS * COLS - 1; i++) {
JButton button = new JButton(String.valueOf(i + 1));
button.setPreferredSize(new Dimension(BUTTON_SIZE, BUTTON_SIZE));
button.addActionListener(this);
puzzleButtonList.add(button);
}
puzzleButtonList.add(null);
Collections.shuffle(puzzleButtonList);
puzzlePanel.removeAll();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int index = i * COLS + j;
JButton button = puzzleButtonList.get(index);
if (button != null) {
puzzlePanel.add(button);
} else {
puzzlePanel.add(new JPanel());
}
}
}
timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
timeCount++;
timeLabel.setText(timeCount + "秒");
}
});
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == resetButton) {
initGame();
timer.start();
} else if (e.getSource() == quitButton) {
System.exit(0);
} else {
JButton button = (JButton) e.getSource();
int index = puzzleButtonList.indexOf(button);
int emptyIndex = puzzleButtonList.indexOf(null);
if (isAdjacent(index, emptyIndex)) {
puzzleButtonList.set(index, null);
puzzleButtonList.set(emptyIndex, button);
puzzlePanel.removeAll();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int position = i * COLS + j;
JButton b = puzzleButtonList.get(position);
if (b != null) {
puzzlePanel.add(b);
} else {
puzzlePanel.add(new JPanel());
}
}
}
stepCount++;
stepLabel.setText("步数:" + stepCount + "步");
if (isWin()) {
timer.stop();
JOptionPane.showMessageDialog(this, "恭喜您,拼图成功!", "游戏结束", JOptionPane.PLAIN_MESSAGE);
}
}
}
}
private boolean isAdjacent(int index1, int index2) {
int row1 = index1 / COLS;
int col1 = index1 % COLS;
int row2 = index2 / COLS;
int col2 = index2 % COLS;
if (row1 == row2 && Math.abs(col1 - col2) == 1) {
return true;
}
if (col1 == col2 && Math.abs(row1 - row2) == 1) {
return true;
}
return false;
}
private boolean isWin() {
for (int i = 0; i < ROWS * COLS - 1; i++) {
JButton button = puzzleButtonList.get(i);
if (button == null || !button.getText().equals(String.valueOf(i + 1))) {
return false;
}
}
return true;
}
public static void main(String[] args) {
new PuzzleGame();
}
}
```
这个示例代码实现了一个3x3的拼图游戏界面,包括拼图块的随机排列、计时、计步等功能。如果你想要实现更大的拼图游戏,可以根据这个示例代码进行扩展。
相关推荐
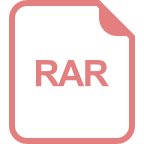
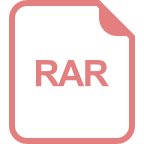














