用C语言编写文件复制程序。分别使用系统调用read (fd,buf,nbytes)、write(fd, buf,nbytes)和文件的库函数fread(buf,size,nitems,fp)、fwrite(buf,size,nitems,fp),编写一个文件复制程序。
时间: 2024-02-20 10:58:32 浏览: 63
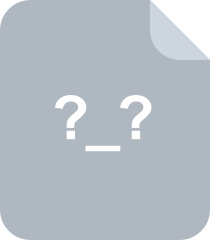
c语言 文件复制
使用系统调用read()和write()函数来实现文件复制程序:
```c
#include <unistd.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
if (argc < 3) {
fprintf(stderr, "Usage: %s <source> <destination>\n", argv[0]);
exit(1);
}
int source_fd = open(argv[1], O_RDONLY);
if (source_fd == -1) {
perror("Failed to open source file");
exit(1);
}
int dest_fd = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH);
if (dest_fd == -1) {
perror("Failed to open destination file");
exit(1);
}
char buffer[BUFFER_SIZE];
ssize_t bytes_read;
while ((bytes_read = read(source_fd, buffer, BUFFER_SIZE)) > 0) {
ssize_t bytes_written = write(dest_fd, buffer, bytes_read);
if (bytes_written != bytes_read) {
perror("Write error");
exit(1);
}
}
if (bytes_read == -1) {
perror("Read error");
exit(1);
}
if (close(source_fd) == -1) {
perror("Failed to close source file");
exit(1);
}
if (close(dest_fd) == -1) {
perror("Failed to close destination file");
exit(1);
}
return 0;
}
```
使用文件的库函数fread()和fwrite()来实现文件复制程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
if (argc < 3) {
fprintf(stderr, "Usage: %s <source> <destination>\n", argv[0]);
exit(1);
}
FILE *source_file = fopen(argv[1], "rb");
if (source_file == NULL) {
perror("Failed to open source file");
exit(1);
}
FILE *dest_file = fopen(argv[2], "wb");
if (dest_file == NULL) {
perror("Failed to open destination file");
exit(1);
}
char buffer[BUFFER_SIZE];
size_t items_read;
while ((items_read = fread(buffer, sizeof(char), BUFFER_SIZE, source_file)) > 0) {
size_t items_written = fwrite(buffer, sizeof(char), items_read, dest_file);
if (items_written != items_read) {
perror("Write error");
exit(1);
}
}
if (ferror(source_file)) {
perror("Read error");
exit(1);
}
if (fclose(source_file) != 0) {
perror("Failed to close source file");
exit(1);
}
if (fclose(dest_file) != 0) {
perror("Failed to close destination file");
exit(1);
}
return 0;
}
```
阅读全文
相关推荐
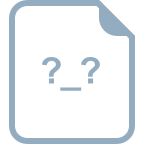
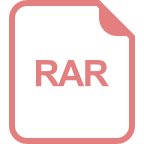







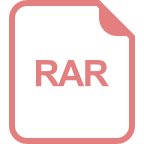
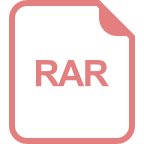
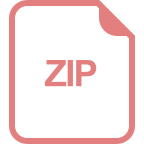