C++ 如何自定义QGraphicsItem
时间: 2024-01-24 14:42:02 浏览: 27
如果你想在 Qt 的 QGraphicsScene 中添加自定义的图形元素,你需要继承 QGraphicsItem 类并实现以下几个函数:
1. boundingRect():返回该图形元素的边界矩形。
2. paint():用于绘制图形元素。
3. shape():返回该图形元素的形状,用于图形元素的事件处理。
4. 要实现自定义的图形元素的交互,你可能还需要重新实现一些 QGraphicsItem 的虚函数,如 mousePressEvent()、mouseMoveEvent()、mouseReleaseEvent()、hoverEnterEvent() 等。
下面是一个继承 QGraphicsItem 的简单例子:
```cpp
class MyItem : public QGraphicsItem
{
public:
MyItem(QGraphicsItem *parent = nullptr);
QRectF boundingRect() const override;
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override;
QPainterPath shape() const override;
protected:
void mousePressEvent(QGraphicsSceneMouseEvent *event) override;
void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override;
void mouseReleaseEvent(QGraphicsSceneMouseEvent *event) override;
private:
QPointF m_pos;
qreal m_radius;
};
MyItem::MyItem(QGraphicsItem *parent)
: QGraphicsItem(parent), m_radius(30)
{
setFlag(ItemIsMovable, true);
}
QRectF MyItem::boundingRect() const
{
return QRectF(-m_radius, -m_radius, m_radius * 2, m_radius * 2);
}
void MyItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
painter->setBrush(Qt::red);
painter->drawEllipse(boundingRect());
}
QPainterPath MyItem::shape() const
{
QPainterPath path;
path.addEllipse(boundingRect());
return path;
}
void MyItem::mousePressEvent(QGraphicsSceneMouseEvent *event)
{
m_pos = event->pos();
}
void MyItem::mouseMoveEvent(QGraphicsSceneMouseEvent *event)
{
QPointF delta = event->pos() - m_pos;
moveBy(delta.x(), delta.y());
m_pos = event->pos();
}
void MyItem::mouseReleaseEvent(QGraphicsSceneMouseEvent *event)
{
Q_UNUSED(event);
}
```
在这个例子中,我们定义了一个圆形的图形元素,并实现了它的 boundingRect()、paint() 和 shape() 函数,以及三个鼠标事件处理函数。在 paint() 函数中,我们使用 QPainter 对象绘制了一个红色的圆形,boundingRect() 函数指定了该图形元素的边界矩形,shape() 函数返回了该圆形的形状,用于事件处理。在鼠标事件处理函数中,我们实现了该图形元素的移动。
相关推荐
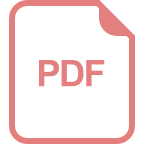
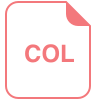













